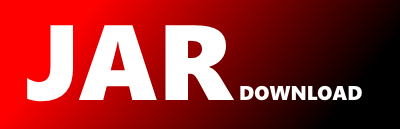
org.infinispan.transaction.xa.CacheTransaction Maven / Gradle / Ivy
package org.infinispan.transaction.xa;
import org.infinispan.commands.write.WriteCommand;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.container.versioning.EntryVersion;
import org.infinispan.container.versioning.EntryVersionsMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Defines the state a infinispan transaction should have.
*
* @author [email protected]
* @since 4.0
*/
public interface CacheTransaction {
/**
* Returns the transaction identifier.
*/
GlobalTransaction getGlobalTransaction();
/**
* Returns the modifications visible within the current transaction. Any modifications using Flag#CACHE_MODE_LOCAL are excluded.
* The returned list is never null.
*/
List getModifications();
/**
* Returns all the modifications visible within the current transaction, including those using Flag#CACHE_MODE_LOCAL.
* The returned list is never null.
*/
List getAllModifications();
/**
* Checks if a modification of the given class (or subclass) is present in this transaction. Any modifications using Flag#CACHE_MODE_LOCAL are ignored.
*
* @param modificationClass the modification type to look for
* @return true if found, false otherwise
*/
boolean hasModification(Class> modificationClass);
CacheEntry lookupEntry(Object key);
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy