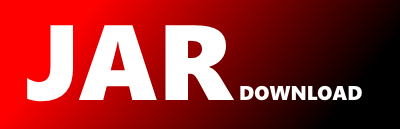
org.infinispan.util.concurrent.locks.containers.AbstractStripedLockContainer Maven / Gradle / Ivy
package org.infinispan.util.concurrent.locks.containers;
import net.jcip.annotations.ThreadSafe;
import org.infinispan.commons.equivalence.Equivalence;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.Lock;
/**
* A container for locks. Used with lock striping.
*
* @author Manik Surtani ([email protected])
* @since 4.0
*/
@ThreadSafe
public abstract class AbstractStripedLockContainer extends AbstractLockContainer {
private int lockSegmentMask;
private int lockSegmentShift;
private final Equivalence
© 2015 - 2025 Weber Informatics LLC | Privacy Policy