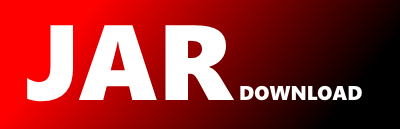
org.infinispan.configuration.cache.IndexingConfigurationBuilder Maven / Gradle / Ivy
package org.infinispan.configuration.cache;
import java.util.Map;
import java.util.Properties;
import org.infinispan.commons.configuration.Builder;
import org.infinispan.commons.util.TypedProperties;
import org.infinispan.commons.util.Util;
import org.infinispan.configuration.global.GlobalConfiguration;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
/**
* Configures indexing of entries in the cache for searching.
*/
public class IndexingConfigurationBuilder extends AbstractConfigurationChildBuilder implements Builder{
private static final Log log = LogFactory.getLog(IndexingConfigurationBuilder.class);
private Properties properties = new Properties();
private Index index = Index.NONE;
private boolean autoConfig;
IndexingConfigurationBuilder(ConfigurationBuilder builder) {
super(builder);
}
/**
* Enable indexing
* @deprecated Use {@link #index(Index)} instead
*/
@Deprecated
public IndexingConfigurationBuilder enable() {
if (this.index == Index.NONE)
this.index = Index.ALL;
return this;
}
/**
* Disable indexing
* @deprecated Use {@link #index(Index)} instead
*/
@Deprecated
public IndexingConfigurationBuilder disable() {
this.index = Index.NONE;
return this;
}
/**
* Enable or disable indexing
* @deprecated Use {@link #index(Index)} instead
*/
@Deprecated
public IndexingConfigurationBuilder enabled(boolean enabled) {
if (this.index == Index.NONE & enabled)
this.index = Index.ALL;
else if (!enabled)
this.index = Index.NONE;
return this;
}
boolean enabled() {
return index.isEnabled();
}
/**
* If true, only index changes made locally, ignoring remote changes. This is useful if indexes
* are shared across a cluster to prevent redundant indexing of updates.
* @deprecated Use {@link #index(Index)} instead
*/
@Deprecated
public IndexingConfigurationBuilder indexLocalOnly(boolean b) {
if (b)
this.index = Index.LOCAL;
return this;
}
boolean indexLocalOnly() {
return this.index.isLocalOnly();
}
/**
*
* Defines a single property. Can be used multiple times to define all needed properties, but the
* full set is overridden by {@link #withProperties(Properties)}.
*
*
* These properties are passed directly to the embedded Hibernate Search engine, so for the
* complete and up to date documentation about available properties refer to the the Hibernate Search
* reference of the version used by Infinispan Query.
*
*
* @see Hibernate
* Search
* @param key Property key
* @param value Property value
* @return this
, for method chaining
*/
public IndexingConfigurationBuilder addProperty(String key, String value) {
this.properties.put(key, value);
return this;
}
/**
*
* Defines a single value. Can be used multiple times to define all needed property values, but the
* full set is overridden by {@link #withProperties(Properties)}.
*
*
* These properties are passed directly to the embedded Hibernate Search engine, so for the
* complete and up to date documentation about available properties refer to the the Hibernate Search
* reference of the version used by Infinispan Query.
*
*
* @see Hibernate
* Search
* @param key Property key
* @param value Property value
* @return this
, for method chaining
*/
public IndexingConfigurationBuilder setProperty(String key, Object value) {
this.properties.put(key, value);
return this;
}
/**
*
* The Query engine relies on properties for configuration.
*
*
* These properties are passed directly to the embedded Hibernate Search engine, so for the
* complete and up to date documentation about available properties refer to the Hibernate Search
* reference of the version you're using with Infinispan Query.
*
*
* @see Hibernate
* Search
* @param props the properties
* @return this
, for method chaining
*/
public IndexingConfigurationBuilder withProperties(Properties props) {
this.properties = props;
return this;
}
/**
* Indicates indexing mode
*/
public IndexingConfigurationBuilder index(Index index) {
this.index = index;
return this;
}
/**
* When enabled, applies to properties default configurations based on
* the cache type
*
* @param autoConfig boolean
* @return this
, for method chaining
*/
public IndexingConfigurationBuilder autoConfig(boolean autoConfig) {
this.autoConfig = autoConfig;
return this;
}
public boolean autoConfig() {
return autoConfig;
}
@Override
public void validate() {
if (index.isEnabled()) {
//Indexing is not conceptually compatible with Invalidation mode
if (clustering().cacheMode().isInvalidation()) {
throw log.invalidConfigurationIndexingWithInvalidation();
}
}
}
@Override
public void validate(GlobalConfiguration globalConfig) {
if (index.isEnabled()) {
// Check that the query module is on the classpath.
try {
String clazz = "org.infinispan.query.Search";
Util.loadClassStrict( clazz, globalConfig.classLoader() );
} catch (ClassNotFoundException e) {
throw log.invalidConfigurationIndexingWithoutModule();
}
}
}
@Override
public IndexingConfiguration create() {
TypedProperties typedProperties = TypedProperties.toTypedProperties(properties);
if (autoConfig()) {
if (clustering().cacheMode().isDistributed()) {
IndexOverlay.DISTRIBUTED_INFINISPAN.apply(typedProperties);
} else {
IndexOverlay.NON_DISTRIBUTED_FS.apply(typedProperties);
}
}
return new IndexingConfiguration(typedProperties, index, autoConfig);
}
@Override
public IndexingConfigurationBuilder read(IndexingConfiguration template) {
this.index = template.index();
this.properties = new Properties();
TypedProperties templateProperties = template.properties();
if (templateProperties != null) {
for (Map.Entry entry : templateProperties.entrySet()) {
properties.put(entry.getKey(), entry.getValue());
}
}
return this;
}
@Override
public String toString() {
return "IndexingConfigurationBuilder{" +
"index=" + index +
", autoConfig=" + autoConfig +
", properties=" + properties +
'}';
}
}