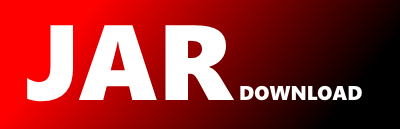
org.infinispan.interceptors.locking.OptimisticLockingInterceptor Maven / Gradle / Ivy
package org.infinispan.interceptors.locking;
import org.infinispan.InvalidCacheUsageException;
import org.infinispan.commands.AbstractVisitor;
import org.infinispan.commands.FlagAffectedCommand;
import org.infinispan.commands.control.LockControlCommand;
import org.infinispan.commands.read.AbstractDataCommand;
import org.infinispan.commands.read.GetCacheEntryCommand;
import org.infinispan.commands.read.GetKeyValueCommand;
import org.infinispan.commands.tx.PrepareCommand;
import org.infinispan.commands.write.ApplyDeltaCommand;
import org.infinispan.commands.write.ClearCommand;
import org.infinispan.commands.write.PutKeyValueCommand;
import org.infinispan.commands.write.PutMapCommand;
import org.infinispan.commands.write.RemoveCommand;
import org.infinispan.commands.write.ReplaceCommand;
import org.infinispan.commands.write.WriteCommand;
import org.infinispan.configuration.cache.CacheMode;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.container.entries.RepeatableReadEntry;
import org.infinispan.context.Flag;
import org.infinispan.context.InvocationContext;
import org.infinispan.context.impl.TxInvocationContext;
import org.infinispan.factories.annotations.Start;
import org.infinispan.util.concurrent.IsolationLevel;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
import java.util.Set;
/**
* Locking interceptor to be used by optimistic transactional caches.
*
* @author Mircea Markus
* @since 5.1
*/
public class OptimisticLockingInterceptor extends AbstractTxLockingInterceptor {
private LockAcquisitionVisitor lockAcquisitionVisitor;
private boolean needToMarkReads;
private static final Log log = LogFactory.getLog(OptimisticLockingInterceptor.class);
@Override
protected Log getLog() {
return log;
}
@Start
public void start() {
if (cacheConfiguration.clustering().cacheMode() == CacheMode.LOCAL &&
cacheConfiguration.locking().writeSkewCheck() &&
cacheConfiguration.locking().isolationLevel() == IsolationLevel.REPEATABLE_READ &&
!cacheConfiguration.unsafe().unreliableReturnValues()) {
lockAcquisitionVisitor = new LocalWriteSkewCheckingLockAcquisitionVisitor();
needToMarkReads = true;
} else {
lockAcquisitionVisitor = new LockAcquisitionVisitor();
needToMarkReads = false;
}
}
private void markKeyAsRead(InvocationContext ctx, AbstractDataCommand command, boolean forceRead) {
if (needToMarkReads && ctx.isInTxScope() &&
(forceRead || !command.hasFlag(Flag.IGNORE_RETURN_VALUES))) {
TxInvocationContext tctx = (TxInvocationContext) ctx;
tctx.getCacheTransaction().addReadKey(command.getKey());
}
}
@Override
public Object visitPrepareCommand(TxInvocationContext ctx, PrepareCommand command) throws Throwable {
if (!command.hasModifications() || command.writesToASingleKey()) {
//optimisation: don't create another LockReorderingVisitor here as it is not needed.
log.trace("Not using lock reordering as we have a single key.");
acquireLocksVisitingCommands(ctx, command);
} else {
Object[] orderedKeys = command.getAffectedKeysToLock(true);
boolean hasClear = orderedKeys == null;
if (hasClear) {
log.trace("Not using lock reordering as the prepare contains a clear command.");
acquireLocksVisitingCommands(ctx, command);
} else {
log.tracef("Using lock reordering, order is: %s", orderedKeys);
acquireAllLocks(ctx, orderedKeys);
}
}
return invokeNextAndCommitIf1Pc(ctx, command);
}
@Override
public Object visitPutKeyValueCommand(InvocationContext ctx, PutKeyValueCommand command) throws Throwable {
if (command.hasFlag(Flag.PUT_FOR_EXTERNAL_READ)) {
// Cache.putForExternalRead() is non-transactional
return super.visitPutKeyValueCommand(ctx, command);
}
try {
markKeyAsRead(ctx, command, command.isConditional());
return invokeNextInterceptor(ctx, command);
} catch (Throwable te) {
throw cleanLocksAndRethrow(ctx, te);
}
}
@Override
public Object visitGetKeyValueCommand(InvocationContext ctx, GetKeyValueCommand command) throws Throwable {
markKeyAsRead(ctx, command, true);
return super.visitGetKeyValueCommand(ctx, command);
}
@Override
public Object visitGetCacheEntryCommand(InvocationContext ctx, GetCacheEntryCommand command) throws Throwable {
markKeyAsRead(ctx, command, true);
return super.visitGetCacheEntryCommand(ctx, command);
}
@Override
public Object visitApplyDeltaCommand(InvocationContext ctx, ApplyDeltaCommand command) throws Throwable {
try {
return invokeNextInterceptor(ctx, command);
} catch (Throwable te) {
throw cleanLocksAndRethrow(ctx, te);
}
}
@Override
public Object visitPutMapCommand(InvocationContext ctx, PutMapCommand command) throws Throwable {
try {
return invokeNextInterceptor(ctx, command);
} catch (Throwable te) {
throw cleanLocksAndRethrow(ctx, te);
}
}
@Override
public Object visitRemoveCommand(InvocationContext ctx, RemoveCommand command) throws Throwable {
try {
// Regardless of whether is conditional so that
// write skews can be detected in both cases.
markKeyAsRead(ctx, command, command.isConditional());
return invokeNextInterceptor(ctx, command);
} catch (Throwable te) {
throw cleanLocksAndRethrow(ctx, te);
}
}
@Override
public Object visitReplaceCommand(InvocationContext ctx, ReplaceCommand command) throws Throwable {
try {
markKeyAsRead(ctx, command, command.isConditional());
return invokeNextInterceptor(ctx, command);
} catch (Throwable te) {
throw cleanLocksAndRethrow(ctx, te);
}
}
@Override
public Object visitClearCommand(InvocationContext ctx, ClearCommand command) throws Throwable {
try {
for (Object key : dataContainer.keySet())
entryFactory.wrapEntryForClear(ctx, key);
return invokeNextInterceptor(ctx, command);
} catch (Throwable te) {
throw cleanLocksAndRethrow(ctx, te);
}
}
@Override
public Object visitLockControlCommand(TxInvocationContext ctx, LockControlCommand command) throws Throwable {
throw new InvalidCacheUsageException(
"Explicit locking is not allowed with optimistic caches!");
}
private class LockAcquisitionVisitor extends AbstractVisitor {
protected void performWriteSkewCheck(TxInvocationContext ctx, Object key) {
// A no-op
}
@Override
public Object visitClearCommand(InvocationContext ctx, ClearCommand command) throws Throwable {
return visitMultiKeyCommand(ctx, command, dataContainer.keySet());
}
@Override
public Object visitPutMapCommand(InvocationContext ctx, PutMapCommand command) throws Throwable {
return visitMultiKeyCommand(ctx, command, command.getMap().keySet());
}
private Object visitMultiKeyCommand(InvocationContext ctx, FlagAffectedCommand command, Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy