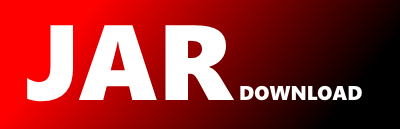
org.infinispan.security.impl.ClusterRoleMapper Maven / Gradle / Ivy
package org.infinispan.security.impl;
import java.security.Principal;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import org.infinispan.commons.util.InfinispanCollections;
import org.infinispan.manager.EmbeddedCacheManager;
import org.infinispan.registry.ClusterRegistry;
import org.infinispan.security.PrincipalRoleMapper;
import org.infinispan.security.PrincipalRoleMapperContext;
/**
* ClusterPrincipalMapper.
*
* @author Tristan Tarrant
* @since 7.0
*/
public class ClusterRoleMapper implements PrincipalRoleMapper {
private EmbeddedCacheManager cacheManager;
private ClusterRegistry, String, Set> clusterRegistry;
@Override
public Set principalToRoles(Principal principal) {
if (clusterRegistry != null) {
return clusterRegistry.get(ClusterRoleMapper.class, principal.getName());
} else {
return Collections.singleton(principal.getName());
}
}
@SuppressWarnings("unchecked")
@Override
public void setContext(PrincipalRoleMapperContext context) {
this.cacheManager = context.getCacheManager();
clusterRegistry = cacheManager.getGlobalComponentRegistry().getComponent(ClusterRegistry.class);
}
public void grant(String roleName, String principalName) {
Set roleSet = clusterRegistry.get(ClusterRoleMapper.class, principalName);
if (roleSet == null) {
roleSet = new HashSet();
}
roleSet.add(roleName);
clusterRegistry.put(ClusterRoleMapper.class, principalName, roleSet);
}
public void deny(String roleName, String principalName) {
Set roleSet = clusterRegistry.get(ClusterRoleMapper.class, principalName);
if (roleSet == null) {
roleSet = new HashSet();
}
roleSet.remove(roleName);
clusterRegistry.put(ClusterRoleMapper.class, principalName, roleSet);
}
public Set list(String principalName) {
Set roleSet = clusterRegistry.get(ClusterRoleMapper.class, principalName);
if (roleSet != null) {
return Collections.unmodifiableSet(roleSet);
} else {
return InfinispanCollections.emptySet();
}
}
public String listAll() {
Set principals = clusterRegistry.keys(ClusterRoleMapper.class);
StringBuilder sb = new StringBuilder();
for(String principal : principals) {
sb.append(list(principal));
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy