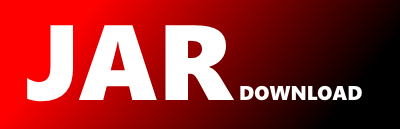
org.infinispan.transaction.impl.TotalOrderRemoteTransactionState Maven / Gradle / Ivy
package org.infinispan.transaction.impl;
import org.infinispan.commons.util.InfinispanCollections;
import org.infinispan.transaction.totalorder.TotalOrderLatch;
import org.infinispan.transaction.xa.GlobalTransaction;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
import java.util.ArrayList;
import java.util.Collection;
import java.util.EnumSet;
import java.util.List;
/**
* Represents a state for a Remote Transaction when the Total Order based protocol is used.
*
* @author Pedro Ruivo
* @since 5.3
*/
public class TotalOrderRemoteTransactionState {
private static final Log log = LogFactory.getLog(TotalOrderRemoteTransactionState.class);
private final EnumSet transactionState;
private final GlobalTransaction globalTransaction;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy