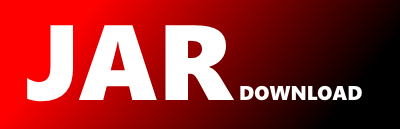
org.infinispan.distribution.ch.ConsistentHash Maven / Gradle / Ivy
package org.infinispan.distribution.ch;
import org.infinispan.commons.hash.Hash;
import org.infinispan.globalstate.ScopedPersistentState;
import org.infinispan.remoting.transport.Address;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* A consistent hash algorithm implementation. Implementations would typically be constructed via a
* {@link ConsistentHashFactory}.
*
* A consistent hash assigns each key a list of owners; the number of owners is defined at creation time,
* but the consistent hash is free to return a smaller or a larger number of owners, depending on
* circumstances, as long as each key has at least one owner.
*
* The first element in the list of owners is the "primary owner". A key will always have a primary owner.
* The other owners are called "backup owners".
*
* This interface gives access to some implementation details of the consistent hash.
*
* Our consistent hashes work by splitting the hash space (the set of possible hash codes) into
* fixed segments and then assigning those segments to nodes dynamically. The number of segments
* is defined at creation time, and the mapping of keys to segments never changes.
* The mapping of segments to nodes can change as the membership of the cache changes.
*
* Normally application code doesn't need to know about this implementation detail, but some
* applications may benefit from the knowledge that all the keys that map to one segment are
* always located on the same server.
*
* @see Non-BlockingStateTransferV2
*
* @author Manik Surtani
* @author [email protected]
* @author Dan Berindei
* @author [email protected]
* @since 4.0
*/
public interface ConsistentHash {
/**
* @return The configured number of owners for each key. Note that {code @getOwners(key)} may return
* a different number of owners.
*/
int getNumOwners();
/**
* @deprecated Since 8.2, the {@code Hash} is optional - replaced in the configuration by the
* {@code KeyPartitioner}
*/
@Deprecated
default Hash getHashFunction() {
throw new UnsupportedOperationException();
}
/**
* @return The actual number of hash space segments. Note that it may not be the same as the number
* of segments passed in at creation time.
*/
int getNumSegments();
/**
* Should return the addresses of the nodes used to create this consistent hash.
*
* @return set of node addresses.
*/
List getMembers();
/**
* Should be equivalent to return the first element of {@link #locateOwners}.
* Useful as a performance optimization, as this is a frequently needed information.
* @param key key to locate
* @return the address of the owner
*/
default Address locatePrimaryOwner(Object key) {
return locatePrimaryOwnerForSegment(getSegment(key));
}
/**
* Finds all the owners of a key. The first element in the returned list is the primary owner.
*
* @param key key to locate
* @return An unmodifiable list of addresses where the key resides.
* Will never be {@code null}, and it will always have at least 1 element.
*/
default List locateOwners(Object key) {
return locateOwnersForSegment(getSegment(key));
}
default Set locateAllOwners(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy