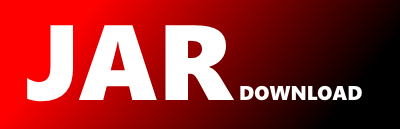
org.infinispan.partitionhandling.impl.PartitionHandlingManager Maven / Gradle / Ivy
package org.infinispan.partitionhandling.impl;
import org.infinispan.commands.write.WriteCommand;
import org.infinispan.container.versioning.EntryVersionsMap;
import org.infinispan.partitionhandling.AvailabilityMode;
import org.infinispan.remoting.transport.Address;
import org.infinispan.topology.CacheTopology;
import org.infinispan.transaction.xa.GlobalTransaction;
import java.util.Collection;
import java.util.List;
/**
* @author Dan Berindei
* @since 7.0
*/
public interface PartitionHandlingManager {
AvailabilityMode getAvailabilityMode();
void setAvailabilityMode(AvailabilityMode availabilityMode);
void checkWrite(Object key);
void checkRead(Object key);
void checkClear();
void checkBulkRead();
@Deprecated //test use only. it can be removed if we update the tests
CacheTopology getLastStableTopology();
/**
* Adds a partially aborted transaction.
*
* The transaction should be registered when it is not sure if the abort happens successfully in all the affected
* nodes.
*
* @param globalTransaction the global transaction.
* @param affectedNodes the nodes involved in the transaction and they must abort the transaction.
* @param lockedKeys the keys locally locked.
* @return {@code true} if the {@link PartitionHandlingManager} will handle it, {@code false} otherwise.
*/
boolean addPartialRollbackTransaction(GlobalTransaction globalTransaction, Collection affectedNodes,
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy