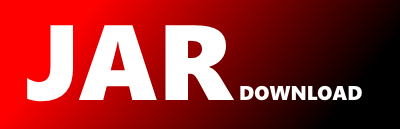
org.jgroups.tests.perf.UUPerf Maven / Gradle / Ivy
package org.jgroups.tests.perf;
import org.jgroups.*;
import org.jgroups.blocks.*;
import org.jgroups.conf.ClassConfigurator;
import org.jgroups.jmx.JmxConfigurator;
import org.jgroups.protocols.UNICAST;
import org.jgroups.protocols.UNICAST2;
import org.jgroups.stack.Protocol;
import org.jgroups.util.*;
import javax.management.MBeanServer;
import java.io.DataInput;
import java.io.DataOutput;
import java.lang.reflect.Method;
import java.nio.ByteBuffer;
import java.text.NumberFormat;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.atomic.AtomicInteger;
/**
* Tests the UNICAST by invoking unicast RPCs from all the nodes to a single node.
* Mimics state transfer in Infinispan.
* @author Dan Berindei
*/
public class UUPerf extends ReceiverAdapter {
private JChannel channel;
private Address local_addr;
private RpcDispatcher disp;
static final String groupname="uuperf";
private final List members=new ArrayList<>();
// ============ configurable properties ==================
private boolean sync=true, oob=true;
private int num_threads=2;
private int num_msgs=1, msg_size=(int)(4.5 * 1000 * 1000);
// =======================================================
private static final Method[] METHODS=new Method[15];
private static final short START=0;
private static final short SET_OOB=1;
private static final short SET_SYNC=2;
private static final short SET_NUM_MSGS=3;
private static final short SET_NUM_THREADS=4;
private static final short SET_MSG_SIZE=5;
private static final short APPLY_STATE=6;
private static final short GET_CONFIG=10;
private final AtomicInteger COUNTER=new AtomicInteger(1);
private byte[] GET_RSP=new byte[msg_size];
static NumberFormat f;
static {
try {
METHODS[START]=UUPerf.class.getMethod("startTest");
METHODS[SET_OOB]=UUPerf.class.getMethod("setOOB",boolean.class);
METHODS[SET_SYNC]=UUPerf.class.getMethod("setSync",boolean.class);
METHODS[SET_NUM_MSGS]=UUPerf.class.getMethod("setNumMessages",int.class);
METHODS[SET_NUM_THREADS]=UUPerf.class.getMethod("setNumThreads",int.class);
METHODS[SET_MSG_SIZE]=UUPerf.class.getMethod("setMessageSize",int.class);
METHODS[APPLY_STATE]=UUPerf.class.getMethod("applyState",byte[].class);
METHODS[GET_CONFIG]=UUPerf.class.getMethod("getConfig");
ClassConfigurator.add((short)12000,Results.class);
f=NumberFormat.getNumberInstance();
f.setGroupingUsed(false);
f.setMinimumFractionDigits(2);
f.setMaximumFractionDigits(2);
}
catch(NoSuchMethodException e) {
throw new RuntimeException(e);
}
}
public void init(String props, String name) throws Throwable {
channel=new JChannel(props);
if(name != null)
channel.setName(name);
disp=new RpcDispatcher(channel,null,this,this);
disp.setMethodLookup(new MethodLookup() {
public Method findMethod(short id) {
return METHODS[id];
}
});
disp.setRequestMarshaller(new CustomMarshaller());
channel.connect(groupname);
local_addr=channel.getAddress();
try {
MBeanServer server=Util.getMBeanServer();
JmxConfigurator.registerChannel(channel,server,"jgroups",channel.getClusterName(),true);
}
catch(Throwable ex) {
System.err.println("registering the channel in JMX failed: " + ex);
}
if(members.size() < 2)
return;
Address coord=members.get(0);
ConfigOptions config=(ConfigOptions)disp.callRemoteMethod(coord,new MethodCall(GET_CONFIG),new RequestOptions(ResponseMode.GET_ALL,5000));
if(config != null) {
this.oob=config.oob;
this.sync=config.sync;
this.num_threads=config.num_threads;
this.num_msgs=config.num_msgs;
this.msg_size=config.msg_size;
System.out.println("Fetched config from " + coord + ": " + config);
}
else
System.err.println("failed to fetch config from " + coord);
}
void stop() {
if(disp != null)
disp.stop();
Util.close(channel);
}
public void viewAccepted(View new_view) {
System.out.println("** view: " + new_view);
members.clear();
members.addAll(new_view.getMembers());
}
// =================================== callbacks ======================================
public Results startTest() throws Throwable {
if(members.indexOf(local_addr) == members.size() - 1) {
System.out.println("This is the joiner, not sending any state");
return new Results(0,0);
}
System.out.println("invoking " + num_msgs + " RPCs of " + Util.printBytes(msg_size) + ", sync=" + sync + ", oob=" + oob);
final AtomicInteger num_msgs_sent=new AtomicInteger(0);
Invoker[] invokers=new Invoker[num_threads];
for(int i=0; i < invokers.length; i++)
invokers[i]=new Invoker(members,num_msgs,num_msgs_sent);
long start=System.currentTimeMillis();
for(Invoker invoker : invokers)
invoker.start();
for(Invoker invoker : invokers) {
invoker.join();
}
long total_time=System.currentTimeMillis() - start;
System.out.println("done (in " + total_time + " ms)");
return new Results(num_msgs_sent.get(),total_time);
}
public void setOOB(boolean oob) {
this.oob=oob;
System.out.println("oob=" + oob);
}
public void setSync(boolean val) {
this.sync=val;
System.out.println("sync=" + sync);
}
public void setNumMessages(int num) {
num_msgs=num;
System.out.println("num_msgs = " + num_msgs);
}
public void setNumThreads(int num) {
num_threads=num;
System.out.println("num_threads = " + num_threads);
}
public void setMessageSize(int num) {
msg_size=num;
System.out.println("msg_size = " + msg_size);
}
public static void applyState(byte[] val) {
System.out.println("-- applyState(): " + Util.printBytes(val.length));
}
public ConfigOptions getConfig() {
return new ConfigOptions(oob,sync,num_threads,num_msgs,msg_size);
}
// ================================= end of callbacks =====================================
public void eventLoop() throws Throwable {
int c;
while(true) {
c=Util.keyPress("[1] Send msgs [2] Print view [3] Print conns " +
"[4] Trash conn [5] Trash all conns" +
"\n[6] Set sender threads (" + num_threads + ") [7] Set num msgs (" + num_msgs + ") " +
"[8] Set msg size (" + Util.printBytes(msg_size) + ")" +
"\n[o] Toggle OOB (" + oob + ") [s] Toggle sync (" + sync + ")" +
"\n[q] Quit\n");
switch(c) {
case -1:
break;
case '1':
try {
startBenchmark();
}
catch(Throwable t) {
System.err.println(t);
}
break;
case '2':
printView();
break;
case '3':
printConnections();
break;
case '4':
removeConnection();
break;
case '5':
removeAllConnections();
break;
case '6':
setSenderThreads();
break;
case '7':
setNumMessages();
break;
case '8':
setMessageSize();
break;
case 'o':
boolean new_value=!oob;
disp.callRemoteMethods(null,new MethodCall(SET_OOB,new_value),RequestOptions.SYNC());
break;
case 's':
boolean new_val=!sync;
disp.callRemoteMethods(null,new MethodCall(SET_SYNC,new_val),RequestOptions.SYNC());
break;
case 'q':
channel.close();
return;
case '\n':
case '\r':
break;
default:
break;
}
}
}
private void printConnections() {
Protocol prot=channel.getProtocolStack().findProtocol(Util.getUnicastProtocols());
if(prot instanceof UNICAST)
System.out.println("connections:\n" + ((UNICAST)prot).printConnections());
else if(prot instanceof UNICAST2)
System.out.println("connections:\n" + ((UNICAST2)prot).printConnections());
}
private void removeConnection() {
Address member=getReceiver();
if(member != null) {
Protocol prot=channel.getProtocolStack().findProtocol(Util.getUnicastProtocols());
if(prot instanceof UNICAST)
((UNICAST)prot).removeConnection(member);
else if(prot instanceof UNICAST2)
((UNICAST2)prot).removeConnection(member);
}
}
private void removeAllConnections() {
Protocol prot=channel.getProtocolStack().findProtocol(Util.getUnicastProtocols());
if(prot instanceof UNICAST)
((UNICAST)prot).removeAllConnections();
else if(prot instanceof UNICAST2)
((UNICAST2)prot).removeAllConnections();
}
/**
* Kicks off the benchmark on all cluster nodes
*/
void startBenchmark() throws Throwable {
RequestOptions options=new RequestOptions(ResponseMode.GET_ALL,0);
options.setFlags(Message.Flag.OOB,Message.Flag.DONT_BUNDLE);
RspList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy