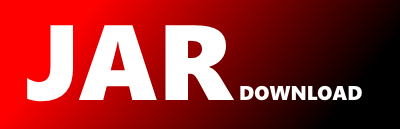
org.infinispan.commands.read.SizeCommand Maven / Gradle / Ivy
package org.infinispan.commands.read;
import org.infinispan.Cache;
import org.infinispan.commands.VisitableCommand;
import org.infinispan.commands.Visitor;
import org.infinispan.commons.util.CloseableIterable;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.context.Flag;
import org.infinispan.context.InvocationContext;
import org.infinispan.filter.AcceptAllKeyValueFilter;
import org.infinispan.filter.NullValueConverter;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
/**
* Command to calculate the size of the cache
*
* @author Manik Surtani ([email protected])
* @author [email protected]
* @author Trustin Lee
* @since 4.0
*/
public class SizeCommand extends AbstractLocalCommand implements VisitableCommand {
private final Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy