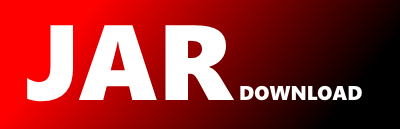
org.infinispan.partitionhandling.impl.PartitionHandlingInterceptor Maven / Gradle / Ivy
package org.infinispan.partitionhandling.impl;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import org.infinispan.commands.DataCommand;
import org.infinispan.commands.LocalFlagAffectedCommand;
import org.infinispan.commands.read.EntrySetCommand;
import org.infinispan.commands.read.GetCacheEntryCommand;
import org.infinispan.commands.read.GetKeyValueCommand;
import org.infinispan.commands.read.GetAllCommand;
import org.infinispan.commands.read.KeySetCommand;
import org.infinispan.commands.tx.CommitCommand;
import org.infinispan.commands.tx.PrepareCommand;
import org.infinispan.commands.write.ApplyDeltaCommand;
import org.infinispan.commands.write.ClearCommand;
import org.infinispan.commands.write.DataWriteCommand;
import org.infinispan.commands.write.PutKeyValueCommand;
import org.infinispan.commands.write.PutMapCommand;
import org.infinispan.commands.write.RemoveCommand;
import org.infinispan.commands.write.ReplaceCommand;
import org.infinispan.commons.util.InfinispanCollections;
import org.infinispan.context.Flag;
import org.infinispan.context.InvocationContext;
import org.infinispan.context.impl.TxInvocationContext;
import org.infinispan.distribution.DistributionManager;
import org.infinispan.factories.annotations.Inject;
import org.infinispan.interceptors.base.CommandInterceptor;
import org.infinispan.partitionhandling.AvailabilityMode;
import org.infinispan.remoting.RpcException;
import org.infinispan.remoting.transport.Transport;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
public class PartitionHandlingInterceptor extends CommandInterceptor {
private static final Log log = LogFactory.getLog(PartitionHandlingInterceptor.class);
private PartitionHandlingManager partitionHandlingManager;
private Transport transport;
private DistributionManager distributionManager;
@Inject
void init(PartitionHandlingManager partitionHandlingManager, Transport transport, DistributionManager distributionManager) {
this.partitionHandlingManager = partitionHandlingManager;
this.transport = transport;
this.distributionManager = distributionManager;
}
private boolean performPartitionCheck(InvocationContext ctx, LocalFlagAffectedCommand command) {
// We always perform partition check if this is a remote command
if (!ctx.isOriginLocal()) {
return true;
}
Set flags = command.getFlags();
return flags == null || !flags.contains(Flag.CACHE_MODE_LOCAL);
}
@Override
public Object visitPutKeyValueCommand(InvocationContext ctx, PutKeyValueCommand command) throws Throwable {
return handleSingleWrite(ctx, command);
}
@Override
public Object visitRemoveCommand(InvocationContext ctx, RemoveCommand command) throws Throwable {
return handleSingleWrite(ctx, command);
}
@Override
public Object visitReplaceCommand(InvocationContext ctx, ReplaceCommand command) throws Throwable {
return handleSingleWrite(ctx, command);
}
protected Object handleSingleWrite(InvocationContext ctx, DataWriteCommand command) throws Throwable {
if (performPartitionCheck(ctx, command)) {
partitionHandlingManager.checkWrite(command.getKey());
}
return handleDefault(ctx, command);
}
@Override
public Object visitPutMapCommand(InvocationContext ctx, PutMapCommand command) throws Throwable {
if (performPartitionCheck(ctx, command)) {
for (Object k : command.getAffectedKeys())
partitionHandlingManager.checkWrite(k);
}
return handleDefault(ctx, command);
}
@Override
public Object visitClearCommand(InvocationContext ctx, ClearCommand command) throws Throwable {
if (performPartitionCheck(ctx, command)) {
partitionHandlingManager.checkClear();
}
return handleDefault(ctx, command);
}
@Override
public Object visitApplyDeltaCommand(InvocationContext ctx, ApplyDeltaCommand command) throws Throwable {
return handleSingleWrite(ctx, command);
}
@Override
public Object visitKeySetCommand(InvocationContext ctx, KeySetCommand command) throws Throwable {
if (performPartitionCheck(ctx, command)) {
partitionHandlingManager.checkBulkRead();
}
return handleDefault(ctx, command);
}
@Override
public Object visitEntrySetCommand(InvocationContext ctx, EntrySetCommand command) throws Throwable {
if (performPartitionCheck(ctx, command)) {
partitionHandlingManager.checkBulkRead();
}
return handleDefault(ctx, command);
}
@Override
public final Object visitGetKeyValueCommand(InvocationContext ctx, GetKeyValueCommand command) throws Throwable {
return handleDataReadCommand(ctx, command);
}
@Override
public final Object visitGetCacheEntryCommand(InvocationContext ctx, GetCacheEntryCommand command) throws Throwable {
return handleDataReadCommand(ctx, command);
}
private Object handleDataReadCommand(InvocationContext ctx, DataCommand command) throws Throwable {
Object key = command.getKey();
Object result;
try {
result = invokeNextInterceptor(ctx, command);
} catch (RpcException e) {
if (performPartitionCheck(ctx, command)) {
// We must have received an AvailabilityException from one of the owners.
// There is no way to verify the cause here, but there isn't any other way to get an invalid get response.
throw getLog().degradedModeKeyUnavailable(key);
} else {
throw e;
}
}
postOperationPartitionCheck(ctx, command, key);
return result;
}
@Override
public Object visitPrepareCommand(TxInvocationContext ctx, PrepareCommand command) throws Throwable {
// Don't send a 2PC prepare at all if the cache is in degraded mode
if (partitionHandlingManager.getAvailabilityMode() != AvailabilityMode.AVAILABLE &&
!command.isOnePhaseCommit() && ctx.hasModifications()) {
for (Object key : ctx.getAffectedKeys()) {
partitionHandlingManager.checkWrite(key);
}
}
Object result = super.visitPrepareCommand(ctx, command);
if (ctx.isOriginLocal()) {
postTxCommandCheck(ctx);
}
return result;
}
@Override
public Object visitCommitCommand(TxInvocationContext ctx, CommitCommand command) throws Throwable {
Object result = super.visitCommitCommand(ctx, command);
if (ctx.isOriginLocal()) {
postTxCommandCheck(ctx);
}
return result;
}
protected void postTxCommandCheck(TxInvocationContext ctx) {
if (partitionHandlingManager.getAvailabilityMode() != AvailabilityMode.AVAILABLE &&
!partitionHandlingManager.isTransactionPartiallyCommitted(ctx.getGlobalTransaction()) &&
ctx.hasModifications()) {
for (Object key : ctx.getAffectedKeys()) {
partitionHandlingManager.checkWrite(key);
}
}
}
private void postOperationPartitionCheck(InvocationContext ctx, DataCommand command, Object key) throws Throwable {
if (performPartitionCheck(ctx, command)) {
// We do the availability check after the read, because the cache may have entered degraded mode
// while we were reading from a remote node.
partitionHandlingManager.checkRead(key);
}
// TODO We can still return a stale value if the other partition stayed active without us and we haven't entered degraded mode yet.
}
@Override
public Object visitGetAllCommand(InvocationContext ctx, GetAllCommand command) throws Throwable {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy