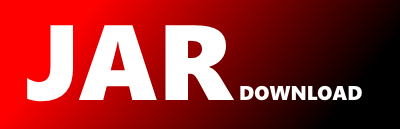
org.infinispan.stream.impl.ClusterStreamManager Maven / Gradle / Ivy
package org.infinispan.stream.impl;
import org.infinispan.CacheStream;
import org.infinispan.distribution.ch.ConsistentHash;
import org.infinispan.remoting.transport.Address;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import java.util.function.Consumer;
import java.util.function.Predicate;
/**
* Manages distribution of various stream operations that are sent to remote nodes. Note usage of any operations
* should always be accompanied with a subsequent call to {@link ClusterStreamManager#forgetOperation(Object)}
* so that the operation is fully released. This is important especially for early terminating operations.
* @param The key type for the underlying cache
*/
public interface ClusterStreamManager {
/**
* A callback that is used for result processing from the remote nodes.
* @param the type of results returned
*/
interface ResultsCallback {
/**
* Called back for intermediate data returned from an operation. This is useful for operations that utilized
* batch fetching such as {@link CacheStream#iterator()}, {@link CacheStream#spliterator()},
* {@link CacheStream#forEach(Consumer)} and {@link CacheStream#toArray()}.
* @param address Which node this data came from
* @param results The results obtained so far.
* @return the segments that completed with some value
*/
Set onIntermediateResult(Address address, R results);
/**
* Essentially the same as {@link ClusterStreamManager.ResultsCallback#onIntermediateResult(Address address, Object)}
* except that this is the last time this callback will be invoked and it tells which segments were completed
* @param address Which node this data came from
* @param results The last batch of results for this operator
*/
void onCompletion(Address address, Set completedSegments, R results);
/**
* Called back when a segment is found to have been lost that is no longer remote
* This method should return as soon as possible and not block in any fashion.
* This method may be invoked concurrently with any of the other methods
* @param segments The segments that were requested but are now local
*/
void onSegmentsLost(Set segments);
}
/**
* Performs the remote stream operation without rehash awareness.
* @param parallelDistribution whether or not parallel distribution is enabled
* @param parallelStream whether or not the stream is paralllel
* @param ch the consistent hash to use when determining segment ownership
* @param segments the segments that this request should utilize
* @param keysToInclude which keys to include in the request
* @param keysToExclude which keys to exclude in the request
* @param includeLoader whether or not to use a loader
* @param operation the actual operation to perform
* @param callback the callback to collect individual node results
* @param earlyTerminatePredicate a predicate to determine if this operation should stop based on intermediate results
* @param the type of response
* @return the operation id to be used for further calls
*/
Object remoteStreamOperation(boolean parallelDistribution, boolean parallelStream, ConsistentHash ch,
Set segments, Set keysToInclude, Map> keysToExclude, boolean includeLoader,
TerminalOperation operation, ResultsCallback callback, Predicate super R> earlyTerminatePredicate);
/**
* Performs the remote stream operation with rehash awareness.
* @param parallelDistribution whether or not parallel distribution is enabled
* @param parallelStream whether or not the stream is paralllel
* @param ch the consistent hash to use when determining segment ownership
* @param segments the segments that this request should utilize
* @param keysToInclude which keys to include in the request
* @param keysToExclude which keys to exclude in the request
* @param includeLoader whether or not to use a loader
* @param operation the actual operation to perform
* @param callback the callback to collect individual node results
* @param earlyTerminatePredicate a predicate to determine if this operation should stop based on intermediate results
* @param the type of response
* @return the operation id to be used for further calls
*/
Object remoteStreamOperationRehashAware(boolean parallelDistribution, boolean parallelStream, ConsistentHash ch,
Set segments, Set keysToInclude, Map> keysToExclude, boolean includeLoader,
TerminalOperation operation, ResultsCallback callback, Predicate super R> earlyTerminatePredicate);
/**
* Key tracking remote operation that doesn't have rehash enabled.
* @param parallelDistribution whether or not parallel distribution is enabled
* @param parallelStream whether or not the stream is paralllel
* @param ch the consistent hash to use when determining segment ownership
* @param segments the segments that this request should utilize
* @param keysToInclude which keys to include in the request
* @param keysToExclude which keys to exclude in the request
* @param includeLoader whether or not to use a loader
* @param operation the actual operation to perform
* @param callback the callback to collect individual node results
* @param the type of response
* @return the operation id to be used for further calls
*/
Object remoteStreamOperation(boolean parallelDistribution, boolean parallelStream, ConsistentHash ch,
Set segments, Set keysToInclude, Map> keysToExclude, boolean includeLoader,
KeyTrackingTerminalOperation operation, ResultsCallback> callback);
/**
* Key tracking remote operation that has rehash enabled
* @param parallelDistribution whether or not parallel distribution is enabled
* @param parallelStream whether or not the stream is paralllel
* @param ch the consistent hash to use when determining segment ownership
* @param segments the segments that this request should utilize
* @param keysToInclude which keys to include in the request
* @param keysToExclude which keys to exclude in the request
* @param includeLoader whether or not to use a loader
* @param operation the actual operation to perform
* @param callback the callback to collect individual node results
* @param the type of response
* @return the operation id to be used for further calls
*/
Object remoteStreamOperationRehashAware(boolean parallelDistribution, boolean parallelStream, ConsistentHash ch,
Set segments, Set keysToInclude,
Map> keysToExclude, boolean includeLoader,
KeyTrackingTerminalOperation operation,
ResultsCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy