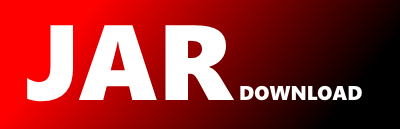
org.infinispan.stream.impl.tx.TxDistributedIntCacheStream Maven / Gradle / Ivy
package org.infinispan.stream.impl.tx;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.context.impl.LocalTxInvocationContext;
import org.infinispan.distribution.ch.ConsistentHash;
import org.infinispan.remoting.transport.Address;
import org.infinispan.stream.impl.AbstractCacheStream;
import org.infinispan.stream.impl.DistributedCacheStream;
import org.infinispan.stream.impl.DistributedDoubleCacheStream;
import org.infinispan.stream.impl.DistributedIntCacheStream;
import org.infinispan.stream.impl.DistributedLongCacheStream;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Int variant of tx cache stream
* @see TxDistributedCacheStream
*/
public class TxDistributedIntCacheStream extends DistributedIntCacheStream {
private final Address localAddress;
private final LocalTxInvocationContext ctx;
private final ConsistentHash hash;
TxDistributedIntCacheStream(AbstractCacheStream stream, Address localAddress, ConsistentHash hash,
LocalTxInvocationContext ctx) {
super(stream);
this.localAddress = localAddress;
this.ctx = ctx;
this.hash = hash;
}
@Override
protected Supplier> supplierForSegments(ConsistentHash ch, Set targetSegments,
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy