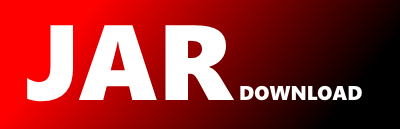
org.infinispan.util.DataContainerRemoveIterator Maven / Gradle / Ivy
package org.infinispan.util;
import org.infinispan.Cache;
import org.infinispan.container.DataContainer;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.container.entries.InternalCacheEntry;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.function.Consumer;
/**
* This is an iterator that will iterate upon the data container. A cache is also provided to be used
* when the remove method on the iterator is invoked. Note that this means it will take part of any
* ongoing transaction if there is one.
*/
public class DataContainerRemoveIterator implements Iterator> {
private final Cache cache;
private final Iterator> dataContainerIterator;
private K previousKey;
public DataContainerRemoveIterator(Cache cache) {
this(cache, cache.getAdvancedCache().getDataContainer());
}
public DataContainerRemoveIterator(Cache cache, DataContainer dataContainer) {
if (cache == null || dataContainer == null) {
throw new NullPointerException();
}
this.cache = cache;
this.dataContainerIterator = dataContainer.iterator();
}
@Override
public boolean hasNext() {
return dataContainerIterator.hasNext();
}
@Override
public CacheEntry next() {
CacheEntry entry = dataContainerIterator.next();
previousKey = entry.getKey();
return entry;
}
@Override
public void remove() {
if (previousKey == null) {
throw new IllegalStateException();
}
cache.remove(previousKey);
previousKey = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy