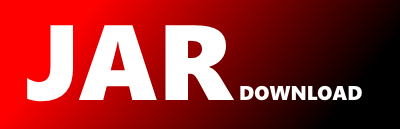
org.jgroups.tests.bla2 Maven / Gradle / Ivy
package org.jgroups.tests;
import org.jgroups.util.Util;
import java.util.LinkedList;
import java.util.List;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.atomic.AtomicInteger;
/**
* @author Bela Ban
* @since x.y
*/
public class bla2 implements Runnable {
protected final BlockingQueue queue=new LinkedBlockingQueue<>(100);
// protected static final int threshold=90;
protected static final int max_bundle_size=10;
protected int count;
protected Thread bundler;
protected final AtomicInteger num=new AtomicInteger(1);
protected final List bundled_msgs=new LinkedList<>();
protected void start() throws Exception {
bundler=new Thread(this, "bundler");
bundler.start();
while(true) {
int c=Util.keyPress(String.format("[1] send single [2] send 5 with sleep [3] send 1000 (queue-size: %d, bundled-msgs size: %d)", queue.size(), bundled_msgs.size()));
switch(c) {
case '1':
int tmp=num.getAndIncrement();
queue.put(tmp);
break;
case '2':
for(int i=0; i < 5; i++) {
tmp=num.getAndIncrement();
queue.put(tmp);
Thread.yield();
}
break;
case '3':
for(int i=0; i < 1000; i++) {
tmp=num.getAndIncrement();
queue.put(tmp);
}
break;
default:
break;
}
}
}
public void run() {
while(true) {
Integer msg=null;
try {
if(count == 0) {
msg=queue.take();
if(msg == null)
continue;
if(count + 1 >= max_bundle_size /*|| queue.size() >= threshold */)
sendBundledMessages();
addMessage(msg);
}
while(null != (msg=queue.poll())) {
if(count + 1 >= max_bundle_size /* || queue.size() >= threshold */)
sendBundledMessages();
addMessage(msg);
}
if(count > 0)
sendBundledMessages();
}
catch(Throwable t) {
}
}
}
protected void addMessage(int num) {
bundled_msgs.add(num);
count++;
}
protected void sendBundledMessages() {
System.out.printf("sending %d messages: %s\n", bundled_msgs.size(), bundled_msgs);
bundled_msgs.clear();
count=0;
}
public static void main(String[] args) throws Exception {
new bla2().start();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy