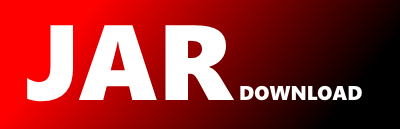
org.jgroups.tests.bla8 Maven / Gradle / Ivy
package org.jgroups.tests;
import org.jgroups.Address;
import org.jgroups.JChannel;
import org.jgroups.blocks.MethodCall;
import org.jgroups.blocks.RequestOptions;
import org.jgroups.blocks.ResponseMode;
import org.jgroups.blocks.RpcDispatcher;
import org.jgroups.util.Util;
import java.lang.reflect.Method;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.atomic.AtomicInteger;
/**
* @author Bela Ban
* @since x.y
*/
public class bla8 {
protected JChannel a;
protected RpcDispatcher disp1;
protected static final int threads=25;
protected static final int num=100000, print=num / 10;
protected static final Method say_method;
protected static final RequestOptions options=new RequestOptions(ResponseMode.GET_ALL, 40000, false, null);
static {
try {
say_method=bla8.class.getDeclaredMethod("sayHello", Address.class);
}
catch(NoSuchMethodException e) {
throw new RuntimeException(e);
}
}
public static String sayHello(Address sender) {
return String.format("hello %s", sender);
}
protected void start(String name) throws Exception {
a=new JChannel("/home/bela/fast.xml").name(name);
disp1=new RpcDispatcher(a, this);
a.connect("demo");
System.out.printf("View is %s\n", a.getView());
while(true) {
Util.keyPress("enter");
AtomicInteger cnt=new AtomicInteger(num);
CountDownLatch latch=new CountDownLatch(threads+1);
MethodCall call=new MethodCall(say_method, a.getAddress());
Invoker[] invokers=new Invoker[threads];
for(int i=0; i < invokers.length; i++) {
invokers[i]=new Invoker(latch, cnt, call);
invokers[i].start();
}
latch.countDown();
for(Invoker inv: invokers)
inv.join();
System.out.println("");
}
}
protected class Invoker extends Thread {
protected final CountDownLatch latch;
protected final AtomicInteger cnt;
protected final MethodCall call;
public Invoker(CountDownLatch latch, AtomicInteger cnt, MethodCall call) {
this.latch=latch;
this.cnt=cnt;
this.call=call;
}
public void run() {
int i;
while((i=cnt.decrementAndGet()) > 0) {
try {
disp1.callRemoteMethods(null, call, options);
if(i % print == 0)
System.out.printf(".");
}
catch(Exception e) {
e.printStackTrace();
}
}
}
}
public static void main(String[] args) throws Exception {
new bla8().start(args[0]);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy