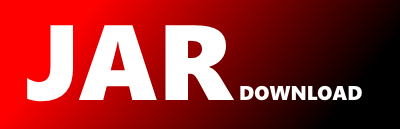
org.jgroups.tests.bla9 Maven / Gradle / Ivy
package org.jgroups.tests;
import org.jgroups.util.Util;
import java.io.IOException;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
import java.util.Set;
/**
* @author Bela Ban
* @since x.y
*/
public class bla9 {
protected ServerSocketChannel srv_ch;
protected Selector sel;
protected void start() throws IOException {
srv_ch=ServerSocketChannel.open();
srv_ch.bind(new InetSocketAddress(InetAddress.getLocalHost(), 7500));
srv_ch.configureBlocking(false);
sel=Selector.open();
srv_ch.register(sel, SelectionKey.OP_ACCEPT);
while(true) {
int num=sel.select();
if(num == 0) continue;
Set keys=sel.selectedKeys();
for(Iterator it=keys.iterator(); it.hasNext();) {
SelectionKey key=it.next();
it.remove();
if(key.isAcceptable())
handleAccept();
if(key.isReadable()) {
handleRead(key);
}
}
}
}
protected void handleAccept() {
SocketChannel client_ch;
try {
client_ch=srv_ch.accept();
client_ch.configureBlocking(false);
client_ch.register(sel, SelectionKey.OP_READ);
}
catch(IOException e) {
e.printStackTrace();
}
}
protected void handleRead(SelectionKey key) {
SocketChannel ch=(SocketChannel)key.channel();
clearSelectionKey(key, SelectionKey.OP_READ);
Reader reader=new Reader(key, ch);
reader.start();
}
protected static int registerSelectionKey(SelectionKey key, int interest_ops) {
if(key == null)
return 0;
key.interestOps(key.interestOps() | interest_ops);
return key.interestOps();
}
protected static int clearSelectionKey(SelectionKey key, int interest_ops) {
if(key == null)
return 0;
key.interestOps(key.interestOps() & ~interest_ops);
return key.interestOps();
}
protected class Reader extends Thread {
protected final SelectionKey key;
protected final SocketChannel ch;
public Reader(SelectionKey key, SocketChannel ch) {
this.key=key;
this.ch=ch;
}
public void run() {
ByteBuffer buf=ByteBuffer.allocate(1);
while(true) {
try {
buf.clear();
int c=ch.read(buf);
if(c == -1) {
Util.close(ch);
break;
}
if(c == 0) {
registerSelectionKey(key, SelectionKey.OP_READ);
key.selector().wakeup();
break;
}
else {
System.out.printf("%c", (char)((ByteBuffer)buf.flip()).get());
Util.sleep(1000);
}
}
catch(IOException e) {
e.printStackTrace();
}
}
System.out.println("");
}
}
public static void main(String[] args) throws Exception {
new bla9().start();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy