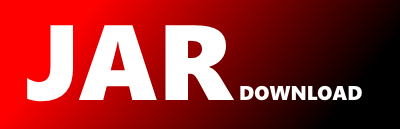
org.jgroups.util.ProcessingQueue Maven / Gradle / Ivy
package org.jgroups.util;
import java.util.Collection;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.locks.ReentrantLock;
/**
* A queue with many producers and consumers. However, only one consumer gets to remove and process elements
* at any given time. This is done through the use of locks.
* @author Bela Ban
* @since 3.5
*/
public class ProcessingQueue {
protected final java.util.Queue queue=new ConcurrentLinkedQueue<>();
protected final ReentrantLock producer_lock=new ReentrantLock(), consumer_lock=new ReentrantLock();
protected int count=0;
protected Handler handler;
public java.util.Queue getQueue() {return queue;}
public int size() {return queue.size();}
public ProcessingQueue setHandler(Handler handler) {this.handler=handler; return this;}
public void add(T element) {
producer_lock.lock();
try {
queue.add(element);
count++;
}
finally {
producer_lock.unlock();
}
process();
}
public boolean retainAll(Collection elements) {
return queue.retainAll(elements);
}
public String toString() {
return queue.toString();
}
protected void process() {
if(consumer_lock.tryLock()) {
try {
while(true) {
T element=queue.poll();
if(element != null) {
if(handler != null) {
try {
handler.handle(element);
}
catch(Throwable t) {
t.printStackTrace(System.err);
}
}
}
producer_lock.lock();
try {
if(count == 0 || count-1 == 0) {
count=0;
consumer_lock.unlock();
return;
}
count--;
}
finally {
producer_lock.unlock();
}
}
}
finally {
if(consumer_lock.isHeldByCurrentThread())
consumer_lock.unlock();
}
}
}
public interface Handler {
void handle(T element);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy