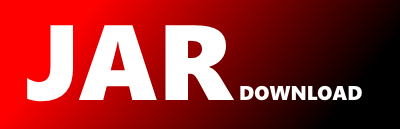
org.infinispan.commands.write.BackupWriteRcpCommand Maven / Gradle / Ivy
package org.infinispan.commands.write;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.concurrent.CompletableFuture;
import org.infinispan.commands.CommandInvocationId;
import org.infinispan.commands.TopologyAffectedCommand;
import org.infinispan.commands.remote.BaseRpcCommand;
import org.infinispan.commons.marshall.MarshallUtil;
import org.infinispan.commons.util.EnumUtil;
import org.infinispan.context.Flag;
import org.infinispan.context.InvocationContext;
import org.infinispan.context.InvocationContextFactory;
import org.infinispan.context.impl.FlagBitSets;
import org.infinispan.interceptors.AsyncInterceptorChain;
import org.infinispan.metadata.Metadata;
import org.infinispan.notifications.cachelistener.CacheNotifier;
import org.infinispan.util.ByteString;
/**
* A command sent from the primary owner to the backup owners of a key with the new update.
*
* This command is only visited by the backups owner and in a remote context. No locks are acquired since it is sent in
* FIFO order, set by {@code sequence}. It can represent a update or remove operation.
*
* @author Pedro Ruivo
* @since 9.0
*/
public class BackupWriteRcpCommand extends BaseRpcCommand implements TopologyAffectedCommand {
public static final byte COMMAND_ID = 61;
private static final Operation[] CACHED_VALUES = Operation.values();
private Operation operation;
private CommandInvocationId commandInvocationId;
private Object key;
private Object value;
private Metadata metadata;
private int topologyId;
private long flags;
private long sequence;
private InvocationContextFactory invocationContextFactory;
private AsyncInterceptorChain interceptorChain;
private CacheNotifier cacheNotifier;
//for org.infinispan.commands.CommandIdUniquenessTest
public BackupWriteRcpCommand() {
super(null);
}
public BackupWriteRcpCommand(ByteString cacheName) {
super(cacheName);
}
private static Operation valueOf(int index) {
return CACHED_VALUES[index];
}
public void setWrite(CommandInvocationId id, Object key, Object value, Metadata metadata, long flags,
int topologyId) {
this.operation = Operation.WRITE;
setCommonAttributes(id, key, flags, topologyId);
this.value = value;
this.metadata = metadata;
}
public void setRemove(CommandInvocationId id, Object key, long flags, int topologyId) {
this.operation = Operation.REMOVE;
setCommonAttributes(id, key, flags, topologyId);
}
public void setRemoveExpired(CommandInvocationId id, Object key, Object value, long flags, int topologyId) {
this.operation = Operation.REMOVE_EXPIRED;
setCommonAttributes(id, key, flags, topologyId);
this.value = value;
}
public void setReplace(CommandInvocationId id, Object key, Object value, Metadata metadata, long flags,
int topologyId) {
this.operation = Operation.REPLACE;
setCommonAttributes(id, key, flags, topologyId);
this.value = value;
this.metadata = metadata;
}
public void init(InvocationContextFactory invocationContextFactory, AsyncInterceptorChain interceptorChain,
CacheNotifier cacheNotifier) {
this.invocationContextFactory = invocationContextFactory;
this.interceptorChain = interceptorChain;
this.cacheNotifier = cacheNotifier;
}
@Override
public CompletableFuture