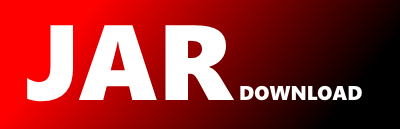
org.infinispan.commons.equivalence.AnyServerEquivalence Maven / Gradle / Ivy
package org.infinispan.commons.equivalence;
import java.util.Arrays;
/**
* AnyServerEquivalence. Works for both objects and byte[]
*
* @author Tristan Tarrant
* @since 5.3
* @deprecated
*/
public class AnyServerEquivalence implements Equivalence
© 2015 - 2025 Weber Informatics LLC | Privacy Policy