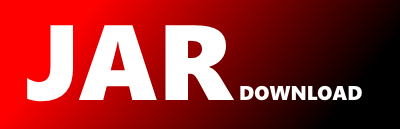
org.infinispan.interceptors.impl.CacheWriterInterceptor Maven / Gradle / Ivy
package org.infinispan.interceptors.impl;
import static org.infinispan.persistence.PersistenceUtil.internalMetadata;
import static org.infinispan.persistence.manager.PersistenceManager.AccessMode.BOTH;
import static org.infinispan.persistence.manager.PersistenceManager.AccessMode.PRIVATE;
import java.util.List;
import java.util.Map;
import java.util.concurrent.atomic.AtomicLong;
import javax.transaction.InvalidTransactionException;
import javax.transaction.SystemException;
import javax.transaction.Transaction;
import javax.transaction.TransactionManager;
import org.infinispan.atomic.impl.AtomicHashMap;
import org.infinispan.commands.AbstractVisitor;
import org.infinispan.commands.FlagAffectedCommand;
import org.infinispan.commands.VisitableCommand;
import org.infinispan.commands.functional.FunctionalCommand;
import org.infinispan.commands.functional.ReadWriteKeyCommand;
import org.infinispan.commands.functional.ReadWriteKeyValueCommand;
import org.infinispan.commands.functional.ReadWriteManyCommand;
import org.infinispan.commands.functional.ReadWriteManyEntriesCommand;
import org.infinispan.commands.functional.WriteOnlyKeyCommand;
import org.infinispan.commands.functional.WriteOnlyKeyValueCommand;
import org.infinispan.commands.functional.WriteOnlyManyCommand;
import org.infinispan.commands.functional.WriteOnlyManyEntriesCommand;
import org.infinispan.commands.tx.CommitCommand;
import org.infinispan.commands.tx.PrepareCommand;
import org.infinispan.commands.write.ApplyDeltaCommand;
import org.infinispan.commands.write.ClearCommand;
import org.infinispan.commands.write.DataWriteCommand;
import org.infinispan.commands.write.PutKeyValueCommand;
import org.infinispan.commands.write.PutMapCommand;
import org.infinispan.commands.write.RemoveCommand;
import org.infinispan.commands.write.ReplaceCommand;
import org.infinispan.commands.write.WriteCommand;
import org.infinispan.commons.api.functional.Param;
import org.infinispan.commons.api.functional.Param.PersistenceMode;
import org.infinispan.commons.marshall.StreamingMarshaller;
import org.infinispan.configuration.cache.PersistenceConfiguration;
import org.infinispan.container.InternalEntryFactory;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.container.entries.DeltaAwareCacheEntry;
import org.infinispan.container.entries.InternalCacheEntry;
import org.infinispan.container.entries.InternalCacheValue;
import org.infinispan.container.versioning.EntryVersion;
import org.infinispan.container.versioning.EntryVersionsMap;
import org.infinispan.context.Flag;
import org.infinispan.context.InvocationContext;
import org.infinispan.context.impl.FlagBitSets;
import org.infinispan.context.impl.TxInvocationContext;
import org.infinispan.factories.annotations.Inject;
import org.infinispan.factories.annotations.Start;
import org.infinispan.interceptors.BasicInvocationStage;
import org.infinispan.jmx.annotations.MBean;
import org.infinispan.jmx.annotations.ManagedAttribute;
import org.infinispan.jmx.annotations.ManagedOperation;
import org.infinispan.jmx.annotations.MeasurementType;
import org.infinispan.marshall.core.MarshalledEntryImpl;
import org.infinispan.metadata.EmbeddedMetadata;
import org.infinispan.metadata.Metadata;
import org.infinispan.persistence.manager.PersistenceManager;
import org.infinispan.transaction.xa.GlobalTransaction;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
/**
* Writes modifications back to the store on the way out: stores modifications back through the CacheLoader, either
* after each method call (no TXs), or at TX commit.
*
* Only used for LOCAL and INVALIDATION caches.
*
* @author Bela Ban
* @author Dan Berindei
* @author Mircea Markus
* @since 9.0
*/
@MBean(objectName = "CacheStore", description = "Component that handles storing of entries to a CacheStore from memory.")
public class CacheWriterInterceptor extends JmxStatsCommandInterceptor {
private final boolean trace = getLog().isTraceEnabled();
PersistenceConfiguration loaderConfig = null;
final AtomicLong cacheStores = new AtomicLong(0);
protected PersistenceManager persistenceManager;
private InternalEntryFactory entryFactory;
private TransactionManager transactionManager;
private StreamingMarshaller marshaller;
private static final Log log = LogFactory.getLog(CacheWriterInterceptor.class);
protected Log getLog() {
return log;
}
@Inject
protected void init(PersistenceManager pm, InternalEntryFactory entryFactory, TransactionManager transactionManager,
StreamingMarshaller marshaller) {
this.persistenceManager = pm;
this.entryFactory = entryFactory;
this.transactionManager = transactionManager;
this.marshaller = marshaller;
}
@Start(priority = 15)
protected void start() {
this.setStatisticsEnabled(cacheConfiguration.jmxStatistics().enabled());
loaderConfig = cacheConfiguration.persistence();
}
@Override
public BasicInvocationStage visitCommitCommand(TxInvocationContext ctx, CommitCommand command) throws Throwable {
commitCommand(ctx);
return invokeNext(ctx, command);
}
@Override
public BasicInvocationStage visitPrepareCommand(TxInvocationContext ctx, PrepareCommand command) throws Throwable {
if (command.isOnePhaseCommit()) {
commitCommand(ctx);
}
return invokeNext(ctx, command);
}
protected void commitCommand(TxInvocationContext ctx) throws Throwable {
if (!ctx.getCacheTransaction().getAllModifications().isEmpty()) {
// this is a commit call.
GlobalTransaction tx = ctx.getGlobalTransaction();
if (trace) getLog().tracef("Calling loader.commit() for transaction %s", tx);
Transaction xaTx = null;
try {
xaTx = suspendRunningTx(ctx);
store(ctx);
} finally {
resumeRunningTx(xaTx);
}
} else {
if (trace) getLog().trace("Commit called with no modifications; ignoring.");
}
}
private void resumeRunningTx(Transaction xaTx) throws InvalidTransactionException, SystemException {
if (transactionManager != null && xaTx != null) {
transactionManager.resume(xaTx);
}
}
private Transaction suspendRunningTx(TxInvocationContext ctx) throws SystemException {
Transaction xaTx = null;
if (transactionManager != null) {
xaTx = transactionManager.suspend();
if (xaTx != null && !ctx.isOriginLocal())
throw new IllegalStateException("It is only possible to be in the context of an JRA transaction in the local node.");
}
return xaTx;
}
@Override
public BasicInvocationStage visitRemoveCommand(InvocationContext ctx, RemoveCommand command) throws Throwable {
return invokeNext(ctx, command).thenAccept((rCtx, rCommand, rv) -> {
RemoveCommand removeCommand = (RemoveCommand) rCommand;
if (!isStoreEnabled(removeCommand) || rCtx.isInTxScope() || !removeCommand.isSuccessful()) return;
if (!isProperWriter(rCtx, removeCommand, removeCommand.getKey())) return;
Object key = removeCommand.getKey();
boolean resp = persistenceManager.deleteFromAllStores(key, BOTH);
if (trace)
getLog().tracef("Removed entry under key %s and got response %s from CacheStore", key, resp);
});
}
@Override
public BasicInvocationStage visitClearCommand(InvocationContext ctx, ClearCommand command) throws Throwable {
if (isStoreEnabled(command) && !ctx.isInTxScope())
persistenceManager.clearAllStores(ctx.isOriginLocal() ? BOTH : PRIVATE);
return invokeNext(ctx, command);
}
@Override
public BasicInvocationStage visitPutKeyValueCommand(InvocationContext ctx, PutKeyValueCommand command) throws Throwable {
return invokeNext(ctx, command).thenAccept((rCtx, rCommand, rv) -> {
PutKeyValueCommand putKeyValueCommand = (PutKeyValueCommand) rCommand;
if (!isStoreEnabled(putKeyValueCommand) || rCtx.isInTxScope() || !putKeyValueCommand.isSuccessful())
return;
if (!isProperWriter(rCtx, putKeyValueCommand, putKeyValueCommand.getKey()))
return;
Object key = putKeyValueCommand.getKey();
storeEntry(rCtx, key, putKeyValueCommand);
if (getStatisticsEnabled())
cacheStores.incrementAndGet();
});
}
@Override
public BasicInvocationStage visitReplaceCommand(InvocationContext ctx, ReplaceCommand command) throws Throwable {
return invokeNext(ctx, command).thenAccept((rCtx, rCommand, rv) -> {
ReplaceCommand replaceCommand = (ReplaceCommand) rCommand;
if (!isStoreEnabled(replaceCommand) || rCtx.isInTxScope() || !replaceCommand.isSuccessful())
return;
if (!isProperWriter(rCtx, replaceCommand, replaceCommand.getKey()))
return;
Object key = replaceCommand.getKey();
storeEntry(rCtx, key, replaceCommand);
if (getStatisticsEnabled())
cacheStores.incrementAndGet();
});
}
@Override
public BasicInvocationStage visitPutMapCommand(InvocationContext ctx, PutMapCommand command) throws Throwable {
return invokeNext(ctx, command).thenAccept((rCtx, rCommand, rv) -> {
PutMapCommand putMapCommand = (PutMapCommand) rCommand;
if (!isStoreEnabled(putMapCommand) || rCtx.isInTxScope())
return;
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy