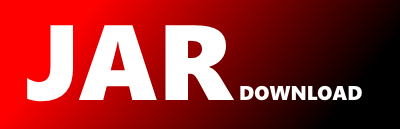
org.infinispan.interceptors.impl.DistCacheWriterInterceptor Maven / Gradle / Ivy
package org.infinispan.interceptors.impl;
import static org.infinispan.commons.util.Util.toStr;
import static org.infinispan.persistence.manager.PersistenceManager.AccessMode.BOTH;
import static org.infinispan.persistence.manager.PersistenceManager.AccessMode.PRIVATE;
import java.util.Map;
import org.infinispan.commands.FlagAffectedCommand;
import org.infinispan.commands.write.PutKeyValueCommand;
import org.infinispan.commands.write.PutMapCommand;
import org.infinispan.commands.write.RemoveCommand;
import org.infinispan.commands.write.ReplaceCommand;
import org.infinispan.context.InvocationContext;
import org.infinispan.context.impl.FlagBitSets;
import org.infinispan.distribution.DistributionManager;
import org.infinispan.factories.annotations.Inject;
import org.infinispan.factories.annotations.Start;
import org.infinispan.interceptors.BasicInvocationStage;
import org.infinispan.interceptors.locking.ClusteringDependentLogic;
import org.infinispan.remoting.transport.Address;
import org.infinispan.remoting.transport.Transport;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
/**
* Cache store interceptor specific for the distribution and replication cache modes.
*
* If the cache store is shared, only the primary owner of the key writes to the cache store.
* If the cache store is not shared, every owner of a key writes to the cache store.
* In non-tx caches, if the originator is an owner, the command is executed there twice. The first time,
* ({@code isOriginLocal() == true}) we don't write anything to the cache store; the second time,
* the normal rules apply.
* For clear operations, either only the originator of the command clears the cache store (if it is
* shared), or every node clears its cache store (if it is not shared). Note that in non-tx caches, this
* happens without holding a lock on the primary owner of all the keys.
*
* @author Galder Zamarreño
* @author Dan Berindei
* @since 9.0
*/
public class DistCacheWriterInterceptor extends CacheWriterInterceptor {
private DistributionManager dm;
private Transport transport;
private Address address;
private static final Log log = LogFactory.getLog(DistCacheWriterInterceptor.class);
private boolean isUsingLockDelegation;
private ClusteringDependentLogic cdl;
@Override
protected Log getLog() {
return log;
}
@Inject
public void inject(DistributionManager dm, Transport transport, ClusteringDependentLogic cdl) {
this.dm = dm;
this.transport = transport;
this.cdl = cdl;
}
@Start(priority = 25) // after the distribution manager!
@SuppressWarnings("unused")
private void setAddress() {
this.address = transport.getAddress();
this.isUsingLockDelegation = !cacheConfiguration.transaction().transactionMode().isTransactional();
}
// ---- WRITE commands
@Override
public BasicInvocationStage visitPutKeyValueCommand(InvocationContext ctx, PutKeyValueCommand command) throws Throwable {
return invokeNext(ctx, command).thenApply((rCtx, rCommand, rv) -> {
PutKeyValueCommand putKeyValueCommand = (PutKeyValueCommand) rCommand;
Object key = putKeyValueCommand.getKey();
if (!isStoreEnabled(putKeyValueCommand) || rCtx.isInTxScope() || !putKeyValueCommand.isSuccessful())
return rv;
if (!isProperWriter(rCtx, putKeyValueCommand, putKeyValueCommand.getKey()))
return rv;
storeEntry(rCtx, key, putKeyValueCommand);
if (getStatisticsEnabled())
cacheStores.incrementAndGet();
return rv;
});
}
@Override
public BasicInvocationStage visitPutMapCommand(InvocationContext ctx, PutMapCommand command) throws Throwable {
return invokeNext(ctx, command).thenApply((rCtx, rCommand, rv) -> {
PutMapCommand putMapCommand = (PutMapCommand) rCommand;
if (!isStoreEnabled(putMapCommand) || rCtx.isInTxScope())
return rv;
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy