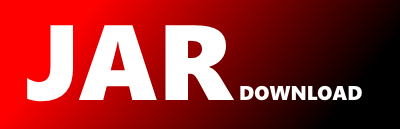
org.infinispan.interceptors.locking.NonTransactionalLockingInterceptor Maven / Gradle / Ivy
package org.infinispan.interceptors.locking;
import java.util.Collection;
import java.util.function.Predicate;
import org.infinispan.InvalidCacheUsageException;
import org.infinispan.commands.DataCommand;
import org.infinispan.commands.FlagAffectedCommand;
import org.infinispan.commands.read.GetAllCommand;
import org.infinispan.commands.write.DataWriteCommand;
import org.infinispan.context.InvocationContext;
import org.infinispan.interceptors.BasicInvocationStage;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
/**
* Locking interceptor to be used for non-transactional caches.
*
* @author Mircea Markus
*/
public class NonTransactionalLockingInterceptor extends AbstractLockingInterceptor {
private static final Log log = LogFactory.getLog(NonTransactionalLockingInterceptor.class);
private final Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy