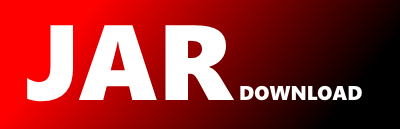
org.infinispan.transaction.impl.RemoteTransaction Maven / Gradle / Ivy
package org.infinispan.transaction.impl;
import static org.infinispan.commons.util.Util.toStr;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedList;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.atomic.AtomicReference;
import org.infinispan.commands.write.WriteCommand;
import org.infinispan.commons.util.CollectionFactory;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.context.Flag;
import org.infinispan.transaction.xa.GlobalTransaction;
import org.infinispan.transaction.xa.InvalidTransactionException;
import org.infinispan.util.concurrent.CompletableFutures;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
/**
* Defines the state of a remotely originated transaction.
*
* @author [email protected]
* @since 4.0
*/
public class RemoteTransaction extends AbstractCacheTransaction implements Cloneable {
private static final Log log = LogFactory.getLog(RemoteTransaction.class);
private static final boolean trace = log.isTraceEnabled();
private static final CompletableFuture INITIAL_FUTURE = CompletableFutures.completedNull();
/**
* This int should be set to the highest topology id for transactions received via state transfer. During state
* transfer we do not migrate lookedUpEntries to save bandwidth. If lookedUpEntriesTopology is less than the
* topology of the CommitCommand that is received this indicates the preceding PrepareCommand was received by
* previous owner before state transfer or the data has now changed owners and the current owner
* now has to re-execute prepare to populate lookedUpEntries (and acquire the locks).
*/
// Default value of MAX_VALUE basically means it hasn't yet received what topology id this is for the entries
private volatile int lookedUpEntriesTopology = Integer.MAX_VALUE;
private volatile TotalOrderRemoteTransactionState transactionState;
private final Object transactionStateLock = new Object();
private final AtomicReference> synchronization =
new AtomicReference<>(INITIAL_FUTURE);
public RemoteTransaction(WriteCommand[] modifications, GlobalTransaction tx, int topologyId, long txCreationTime) {
super(tx, topologyId, txCreationTime);
this.modifications = modifications == null || modifications.length == 0
? Collections.emptyList()
: Arrays.asList(modifications);
lookedUpEntries = CollectionFactory.makeMap(CollectionFactory.computeCapacity(this.modifications.size()));
}
public RemoteTransaction(GlobalTransaction tx, int topologyId, long txCreationTime) {
super(tx, topologyId, txCreationTime);
this.modifications = new LinkedList<>();
lookedUpEntries = CollectionFactory.makeMap(4);
}
@Override
public void setStateTransferFlag(Flag stateTransferFlag) {
if (getStateTransferFlag() == null && stateTransferFlag == Flag.PUT_FOR_X_SITE_STATE_TRANSFER) {
internalSetStateTransferFlag(stateTransferFlag);
}
}
@Override
public void putLookedUpEntry(Object key, CacheEntry e) {
checkIfRolledBack();
if (trace) {
log.tracef("Adding key %s to tx %s", toStr(key), getGlobalTransaction());
}
lookedUpEntries.put(key, e);
}
@Override
public void putLookedUpEntries(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy