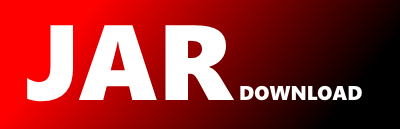
org.infinispan.commands.functional.ReadWriteKeyCommand Maven / Gradle / Ivy
package org.infinispan.commands.functional;
import static org.infinispan.commons.util.Util.toStr;
import static org.infinispan.functional.impl.EntryViews.snapshot;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.function.Function;
import org.infinispan.commands.CommandInvocationId;
import org.infinispan.commands.Visitor;
import org.infinispan.commands.functional.functions.InjectableComponent;
import org.infinispan.commands.write.ValueMatcher;
import org.infinispan.commons.marshall.MarshallUtil;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.context.InvocationContext;
import org.infinispan.context.impl.FlagBitSets;
import org.infinispan.factories.ComponentRegistry;
import org.infinispan.functional.EntryView.ReadWriteEntryView;
import org.infinispan.functional.impl.EntryViews;
import org.infinispan.functional.impl.Params;
// TODO: the command does not carry previous values to backup, so it can cause
// the values on primary and backup owners to diverge in case of topology change
public final class ReadWriteKeyCommand extends AbstractWriteKeyCommand {
public static final byte COMMAND_ID = 50;
private Function, R> f;
public ReadWriteKeyCommand(K key, Function, R> f,
CommandInvocationId id, ValueMatcher valueMatcher, Params params,
ComponentRegistry componentRegistry) {
super(key, valueMatcher, id, params);
this.f = f;
this.init(componentRegistry);
}
public ReadWriteKeyCommand() {
// No-op, for marshalling
}
@Override
public byte getCommandId() {
return COMMAND_ID;
}
@Override
public void writeTo(ObjectOutput output) throws IOException {
output.writeObject(key);
output.writeObject(f);
MarshallUtil.marshallEnum(valueMatcher, output);
Params.writeObject(output, params);
output.writeLong(FlagBitSets.copyWithoutRemotableFlags(getFlagsBitSet()));
CommandInvocationId.writeTo(output, commandInvocationId);
}
@Override
public void readFrom(ObjectInput input) throws IOException, ClassNotFoundException {
key = input.readObject();
f = (Function, R>) input.readObject();
valueMatcher = MarshallUtil.unmarshallEnum(input, ValueMatcher::valueOf);
params = Params.readObject(input);
setFlagsBitSet(input.readLong());
commandInvocationId = CommandInvocationId.readFrom(input);
}
@Override
public boolean isConditional() {
return true;
}
@Override
public Object perform(InvocationContext ctx) throws Throwable {
// It's not worth looking up the entry if we're never going to apply the change.
if (valueMatcher == ValueMatcher.MATCH_NEVER) {
successful = false;
return null;
}
CacheEntry e = ctx.lookupEntry(key);
// Could be that the key is not local, 'null' is how this is signalled
if (e == null) return null;
R ret = f.apply(EntryViews.readWrite(e));
return snapshot(ret);
}
@Override
public Object acceptVisitor(InvocationContext ctx, Visitor visitor) throws Throwable {
return visitor.visitReadWriteKeyCommand(ctx, this);
}
@Override
public LoadType loadType() {
return LoadType.OWNER;
}
@Override
public Mutation toMutation(K key) {
return new Mutations.ReadWrite<>(f);
}
public Function, R> getFunction() {
return f;
}
@Override
public String toString() {
return "ReadWriteKeyCommand" +
"{ key=" + toStr(key) +
", f=" + f +
", flags=" + printFlags() +
", commandInvocationId=" + commandInvocationId +
", params=" + params +
", valueMatcher=" + valueMatcher +
", successful=" + successful +
"}";
}
public void init(ComponentRegistry componentRegistry) {
if (f instanceof InjectableComponent) {
((InjectableComponent) f).inject(componentRegistry);
}
}
@Override
public final boolean isReturnValueExpected() {
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy