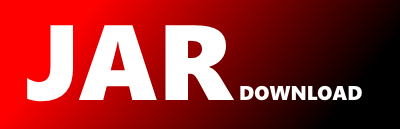
org.infinispan.commands.functional.WriteOnlyManyEntriesCommand Maven / Gradle / Ivy
package org.infinispan.commands.functional;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Collection;
import java.util.Map;
import java.util.function.BiConsumer;
import org.infinispan.commands.CommandInvocationId;
import org.infinispan.commands.Visitor;
import org.infinispan.functional.EntryView.WriteEntryView;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.context.InvocationContext;
import org.infinispan.functional.impl.EntryViews;
import org.infinispan.functional.impl.Params;
public final class WriteOnlyManyEntriesCommand extends AbstractWriteManyCommand {
public static final byte COMMAND_ID = 57;
private Map extends K, ? extends V> entries;
private BiConsumer> f;
public WriteOnlyManyEntriesCommand(Map extends K, ? extends V> entries, BiConsumer> f, Params params, CommandInvocationId commandInvocationId) {
super(commandInvocationId, params);
this.entries = entries;
this.f = f;
}
public WriteOnlyManyEntriesCommand(WriteOnlyManyEntriesCommand command) {
super(command);
this.entries = command.entries;
this.f = command.f;
}
public WriteOnlyManyEntriesCommand() {
}
public Map extends K, ? extends V> getEntries() {
return entries;
}
public void setEntries(Map extends K, ? extends V> entries) {
this.entries = entries;
}
public final WriteOnlyManyEntriesCommand withEntries(Map extends K, ? extends V> entries) {
setEntries(entries);
return this;
}
@Override
public byte getCommandId() {
return COMMAND_ID;
}
@Override
public void writeTo(ObjectOutput output) throws IOException {
CommandInvocationId.writeTo(output, commandInvocationId);
output.writeObject(entries);
output.writeObject(f);
output.writeBoolean(isForwarded);
Params.writeObject(output, params);
output.writeInt(topologyId);
output.writeLong(flags);
}
@Override
public void readFrom(ObjectInput input) throws IOException, ClassNotFoundException {
commandInvocationId = CommandInvocationId.readFrom(input);
entries = (Map extends K, ? extends V>) input.readObject();
f = (BiConsumer>) input.readObject();
isForwarded = input.readBoolean();
params = Params.readObject(input);
topologyId = input.readInt();
flags = input.readLong();
}
@Override
public Object perform(InvocationContext ctx) throws Throwable {
for (Map.Entry extends K, ? extends V> entry : entries.entrySet()) {
CacheEntry cacheEntry = ctx.lookupEntry(entry.getKey());
// Could be that the key is not local, 'null' is how this is signalled
if (cacheEntry == null) {
throw new IllegalStateException();
}
f.accept(entry.getValue(), EntryViews.writeOnly(cacheEntry));
}
return null;
}
@Override
public boolean isReturnValueExpected() {
return false;
}
@Override
public Collection> getAffectedKeys() {
return entries.keySet();
}
@Override
public Object acceptVisitor(InvocationContext ctx, Visitor visitor) throws Throwable {
return visitor.visitWriteOnlyManyEntriesCommand(ctx, this);
}
@Override
public LoadType loadType() {
return LoadType.DONT_LOAD;
}
@Override
public boolean isWriteOnly() {
return true;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("WriteOnlyManyEntriesCommand{");
sb.append("entries=").append(entries);
sb.append(", f=").append(f.getClass().getName());
sb.append(", isForwarded=").append(isForwarded);
sb.append('}');
return sb.toString();
}
@Override
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy