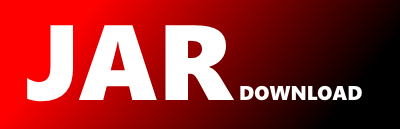
org.infinispan.container.offheap.UnsafeHolder Maven / Gradle / Ivy
package org.infinispan.container.offheap;
import java.lang.reflect.Field;
import java.security.AccessController;
import java.security.PrivilegedAction;
import org.infinispan.commons.CacheException;
import sun.misc.Unsafe;
/**
* @author wburns
* @since 9.0
*/
class UnsafeHolder {
static Unsafe UNSAFE = UnsafeHolder.getUnsafe();
@SuppressWarnings("restriction")
private static Unsafe getUnsafe() {
// attempt to access field Unsafe#theUnsafe
final Object maybeUnsafe = AccessController.doPrivileged((PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy