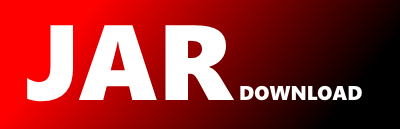
org.infinispan.distribution.group.impl.GroupManagerImpl Maven / Gradle / Ivy
package org.infinispan.distribution.group.impl;
import static org.infinispan.commons.util.ReflectionUtil.invokeAccessibly;
import java.lang.reflect.Method;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.ConcurrentMap;
import org.infinispan.commons.util.CollectionFactory;
import org.infinispan.commons.util.ReflectionUtil;
import org.infinispan.commons.util.Util;
import org.infinispan.distribution.DistributionManager;
import org.infinispan.distribution.group.Group;
import org.infinispan.distribution.group.Grouper;
import org.infinispan.factories.annotations.Inject;
import org.infinispan.remoting.transport.Address;
public class GroupManagerImpl implements GroupManager {
private interface GroupMetadata {
GroupMetadata NONE = instance -> null;
Object getGroup(Object instance);
}
private static class GroupMetadataImpl implements GroupMetadata {
private final Method method;
GroupMetadataImpl(Method method) {
if (method.getParameterTypes().length > 0)
throw new IllegalArgumentException(Util.formatString("@Group method %s must have zero arguments", method));
this.method = method;
}
@Override
public Object getGroup(Object instance) {
Object object;
if (System.getSecurityManager() == null) {
return invokeAccessibly(instance, method, Util.EMPTY_OBJECT_ARRAY);
} else {
return AccessController.doPrivileged((PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy