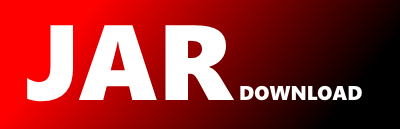
org.infinispan.factories.components.ManageableComponentMetadata Maven / Gradle / Ivy
package org.infinispan.factories.components;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.infinispan.jmx.annotations.MBean;
import org.infinispan.jmx.annotations.ManagedAttribute;
import org.infinispan.jmx.annotations.ManagedOperation;
/**
* A specialization of {@link ComponentMetadata}, this version also includes JMX related metadata, as expressed
* by {@link MBean}, {@link ManagedAttribute} and {@link ManagedOperation} annotations.
*
* @author Manik Surtani
* @since 5.1
*/
public class ManageableComponentMetadata extends ComponentMetadata {
private String jmxObjectName;
private String description;
private Set attributeMetadata;
private Set operationMetadata;
public ManageableComponentMetadata(Class> component, List injectMethods, List startMethods, List stopMethods, boolean global, boolean survivesRestarts, List managedAttributeFields, List managedAttributeMethods, List managedOperationMethods, MBean mbean) {
super(component, injectMethods, startMethods, stopMethods, global, survivesRestarts);
if ((managedAttributeFields != null && !managedAttributeFields.isEmpty()) || (managedAttributeMethods != null && !managedAttributeMethods.isEmpty())) {
attributeMetadata = new HashSet((managedAttributeFields == null ? 0 : managedAttributeFields.size()) + (managedAttributeMethods == null ? 0 : managedAttributeMethods.size()));
if (managedAttributeFields != null) {
for (Field f: managedAttributeFields) attributeMetadata.add(new JmxAttributeMetadata(f));
}
if (managedAttributeMethods != null) {
for (Method m: managedAttributeMethods) attributeMetadata.add(new JmxAttributeMetadata(m));
}
}
if (managedOperationMethods != null && !managedOperationMethods.isEmpty()) {
operationMetadata = new HashSet(managedOperationMethods.size());
for (Method m: managedOperationMethods) operationMetadata.add(new JmxOperationMetadata(m));
}
jmxObjectName = mbean.objectName();
description = mbean.description();
}
public String getJmxObjectName() {
return jmxObjectName;
}
public String getDescription() {
return description;
}
public Set getAttributeMetadata() {
if (attributeMetadata == null) return Collections.emptySet();
return attributeMetadata;
}
public Set getOperationMetadata() {
if (operationMetadata == null) return Collections.emptySet();
return operationMetadata;
}
@Override
public boolean isManageable() {
return true;
}
@Override
public ManageableComponentMetadata toManageableComponentMetadata() {
return this;
}
@Override
public String toString() {
return "ManageableComponentMetadata{" +
"jmxObjectName='" + jmxObjectName + '\'' +
", description='" + description + '\'' +
", attributeMetadata=" + attributeMetadata +
", operationMetadata=" + operationMetadata +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy