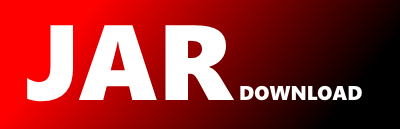
org.infinispan.persistence.support.BatchModification Maven / Gradle / Ivy
package org.infinispan.persistence.support;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.infinispan.marshall.core.MarshalledEntry;
/**
* A simple wrapper class, necessary for Transactional stores, which allows MarshalledEntries and Object keys to be passed
* to a store implementation in order. This class also removes repeated operations on the same key in order to prevent
* redundant operations on the underlying store. For example a tx, {put(1, "Test"); remove(1);}, will be simply written
* to the store as {remove(1);}.
*
* @author Ryan Emerson
*/
public class BatchModification {
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy