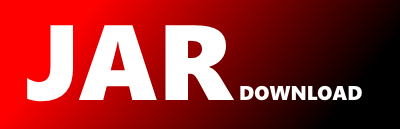
org.jgroups.tests.bla5 Maven / Gradle / Ivy
package org.jgroups.tests;
import org.jgroups.util.Util;
import java.util.concurrent.DelayQueue;
import java.util.concurrent.Delayed;
import java.util.concurrent.TimeUnit;
import java.util.stream.Stream;
/**
* @author Bela Ban
* @since x.y
*/
public class bla5 {
DelayQueue queue=new DelayQueue<>();
public static void main(String[] args) throws Exception {
new bla5().start();
}
private void start() throws InterruptedException {
queue.add(new DelayedInt(1, System.currentTimeMillis() + 5000));
new Thread(() -> {
Util.sleep(1000);
Stream.of(2,3,6,7).forEach(n -> queue.add(new DelayedInt(n, n*1000)));
})
.start();
for(;;) {
DelayedInt el=queue.poll(10000, TimeUnit.MILLISECONDS);
System.out.println("el = " + el);
}
}
protected static class DelayedInt implements Delayed {
protected final int num;
protected final long target_time;
public DelayedInt(int num, long target_time) {
this.num=num;
this.target_time=target_time;
}
public int compareTo(Delayed o) {
DelayedInt other=(DelayedInt)o;
long my_delay=getDelay(TimeUnit.MILLISECONDS), other_delay=other.getDelay(TimeUnit.MILLISECONDS);
return my_delay < other_delay ? -1 : my_delay > other_delay? 1 : 0;
}
public long getDelay(TimeUnit unit) {
long delay=target_time - System.currentTimeMillis();
return unit.convert(delay, TimeUnit.MILLISECONDS);
}
public String toString() {
return String.valueOf(num);
}
}
/* public static void testParseAddresses() throws Exception {
final String input="addrs\n" +
"physical-addrs=1 2 3 4\n" +
"local_addr=ip-172-31-40-246-49335 [ip=172.31.40.246:7900, version=4.0.1.Final (Schiener Berg), cluster=cfg, 16 mbr(s)]\n" +
"addrs=172.31.43.129:7900, 172.31.45.32:7900, 172.31.44.159:7900, 172.31.34.253:7900, 172.31.45.220:7900, 172.31.47.25:7900, 172.31.47.249:7900, 172.31.40.246:7900, 172.31.43.212:7900, 172.31.46.83:7900, 172.31.39.17:7900, 172.31.38.240:7900, 172.31.38.13:7900, 172.31.33.109:7900, 172.31.46.77:7900, 172.31.39.5:7900\n" +
"\n" +
"local_addr=ip-172-31-40-246-49335";
Collection addrs=Probe.parseAddresses(input);
System.out.println("addrs = " + addrs);
}*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy