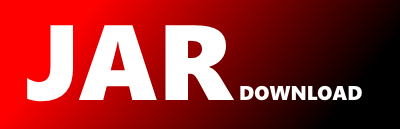
org.infinispan.commons.util.ServiceFinder Maven / Gradle / Ivy
package org.infinispan.commons.util;
import java.util.LinkedHashSet;
import java.util.ServiceLoader;
import java.util.Set;
import org.infinispan.commons.logging.Log;
import org.infinispan.commons.logging.LogFactory;
import org.osgi.framework.BundleContext;
import org.osgi.framework.BundleReference;
import org.osgi.util.tracker.ServiceTracker;
/**
* ServiceFinder is a {@link java.util.ServiceLoader} replacement which understands multiple
* classpaths
*
* @author Tristan Tarrant
* @author Brett Meyer
* @since 6.0
*/
public class ServiceFinder {
private static final Log LOG = LogFactory.getLog(ServiceFinder.class);
public static Set load(Class contract, ClassLoader... loaders) {
Set services = new LinkedHashSet();
if (loaders.length == 0) {
try {
ServiceLoader loadedServices = ServiceLoader.load(contract);
addServices( loadedServices, services );
} catch (Exception e) {
// Ignore
}
}
else {
for (ClassLoader loader : loaders) {
if (loader == null)
continue;
try {
ServiceLoader loadedServices = ServiceLoader.load(contract, loader);
addServices( loadedServices, services );
} catch (Exception e) {
// Ignore
}
}
}
addOsgiServices( contract, services );
if (services.isEmpty()) {
LOG.debugf("No service impls found: %s", contract.getSimpleName());
}
return services;
}
private static void addServices(ServiceLoader loadedServices, Set services) {
if (loadedServices.iterator().hasNext()) {
for (T loadedService : loadedServices) {
LOG.debugf("Loading service impl: %s", loadedService.getClass().getSimpleName());
services.add(loadedService);
}
}
}
private static void addOsgiServices(Class contract, Set services) {
if (!Util.isOSGiContext()) {
return;
}
ClassLoader loader = ServiceFinder.class.getClassLoader();
if ((loader != null) && (loader instanceof org.osgi.framework.BundleReference)) {
final BundleContext bundleContext = ((BundleReference) loader).getBundle().getBundleContext();
final ServiceTracker serviceTracker = new ServiceTracker(bundleContext, contract.getName(),
null);
serviceTracker.open();
try {
final Object[] osgiServices = serviceTracker.getServices();
if (osgiServices != null) {
for (Object osgiService : osgiServices) {
services.add((T) osgiService);
}
}
} catch (Exception e) {
// ignore
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy