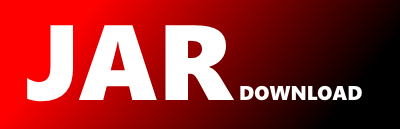
org.infinispan.protostream.config.AnnotationAttributeConfig Maven / Gradle / Ivy
package org.infinispan.protostream.config;
import org.infinispan.protostream.AnnotationMetadataCreator;
import org.infinispan.protostream.descriptors.AnnotatedDescriptor;
import java.util.HashSet;
import java.util.Set;
/**
* @author [email protected]
* @since 2.0
*/
public final class AnnotationAttributeConfig {
public enum AttributeType {
IDENTIFIER, STRING, CHARACTER, BOOLEAN, INT, LONG, FLOAT, DOUBLE, ANNOTATION
}
private final String name;
private final boolean isMultiple;
private final Object defaultValue;
private final AttributeType type;
private final Set allowedValues;
private AnnotationAttributeConfig(String name, boolean isMultiple, Object defaultValue, AttributeType type, Set allowedValues) {
this.name = name;
this.isMultiple = isMultiple;
this.defaultValue = defaultValue;
this.type = type;
this.allowedValues = allowedValues;
}
public String name() {
return name;
}
public boolean multiple() {
return isMultiple;
}
public Object defaultValue() {
return defaultValue;
}
public AttributeType type() {
return type;
}
public Set allowedValues() {
return allowedValues;
}
public static final class Builder {
private final AnnotationConfig.Builder parentBuilder;
private final String name;
private boolean isMultiple;
private Object defaultValue;
private AttributeType type = AttributeType.STRING;
private String[] allowedValues;
Builder(AnnotationConfig.Builder parentBuilder, String name) {
this.parentBuilder = parentBuilder;
this.name = name;
}
public Builder multiple(boolean isMultiple) {
this.isMultiple = isMultiple;
return this;
}
public Builder defaultValue(Object defaultValue) {
if (defaultValue == null) {
throw new IllegalArgumentException("Default value cannot be null");
}
this.defaultValue = defaultValue;
return this;
}
public Builder annotationType(String... allowedAnnotations) {
type = AttributeType.ANNOTATION;
allowedValues = allowedAnnotations;
return this;
}
public Builder identifierType(String... allowedValues) {
type = AttributeType.IDENTIFIER;
this.allowedValues = allowedValues;
return this;
}
public Builder stringType(String... allowedValues) {
type = AttributeType.STRING;
this.allowedValues = allowedValues;
return this;
}
public Builder characterType() {
type = AttributeType.CHARACTER;
allowedValues = null;
return this;
}
public Builder booleanType() {
type = AttributeType.BOOLEAN;
allowedValues = null;
return this;
}
public Builder intType() {
type = AttributeType.INT;
allowedValues = null;
return this;
}
public Builder longType() {
type = AttributeType.LONG;
allowedValues = null;
return this;
}
public Builder floatType() {
type = AttributeType.FLOAT;
allowedValues = null;
return this;
}
public Builder doubleType() {
type = AttributeType.DOUBLE;
allowedValues = null;
return this;
}
public Builder attribute(String name) {
return parentBuilder.attribute(name);
}
public AnnotationConfig.Builder annotationMetadataCreator(AnnotationMetadataCreator, DescriptorType> annotationMetadataCreator) {
return parentBuilder.annotationMetadataCreator(annotationMetadataCreator);
}
AnnotationAttributeConfig buildAnnotationAttributeConfig() {
Set allowedValuesSet = null;
if (allowedValues != null && allowedValues.length != 0) {
switch (type) {
case ANNOTATION:
case IDENTIFIER:
case STRING:
allowedValuesSet = new HashSet(allowedValues.length);
for (String v : allowedValues) {
allowedValuesSet.add(v);
}
break;
default:
throw new IllegalArgumentException("The type of attribute '" + name + "' does not support a set of allowed values");
}
}
if (defaultValue != null) {
switch (type) {
case ANNOTATION:
throw new IllegalArgumentException("The type of attribute '" + name + "' does not allow a default value.");
case STRING:
case IDENTIFIER:
if (!(defaultValue instanceof String)) {
throw new IllegalArgumentException("Illegal default value type for attribute '" + name + "'. String expected.");
}
break;
case CHARACTER:
if (!(defaultValue instanceof Character)) {
throw new IllegalArgumentException("Illegal default value type for attribute '" + name + "'. Character expected.");
}
break;
case BOOLEAN:
if (!(defaultValue instanceof Boolean)) {
throw new IllegalArgumentException("Illegal default value type for attribute '" + name + "'. Boolean expected.");
}
break;
case INT:
if (!(defaultValue instanceof Integer)) {
throw new IllegalArgumentException("Illegal default value type for attribute '" + name + "'. Integer expected.");
}
break;
case LONG:
if (!(defaultValue instanceof Long)) {
throw new IllegalArgumentException("Illegal default value type for attribute '" + name + "'. Long expected.");
}
break;
case FLOAT:
if (!(defaultValue instanceof Float)) {
throw new IllegalArgumentException("Illegal default value type for attribute '" + name + "'. Float expected.");
}
break;
case DOUBLE:
if (!(defaultValue instanceof Double)) {
throw new IllegalArgumentException("Illegal default value type for attribute '" + name + "'. Double expected.");
}
break;
}
}
return new AnnotationAttributeConfig(name, isMultiple, defaultValue, type, allowedValuesSet);
}
public Configuration build() {
return parentBuilder.build();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy