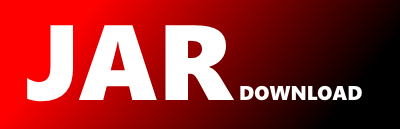
org.infinispan.protostream.config.Configuration Maven / Gradle / Ivy
package org.infinispan.protostream.config;
import org.infinispan.protostream.AnnotationMetadataCreator;
import org.infinispan.protostream.descriptors.AnnotationElement;
import org.infinispan.protostream.descriptors.Descriptor;
import org.infinispan.protostream.descriptors.EnumDescriptor;
import org.infinispan.protostream.descriptors.FieldDescriptor;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* @author [email protected]
* @since 2.0
*/
public final class Configuration {
public static final String TYPE_ID_ANNOTATION = "TypeId";
private final boolean logOutOfSequenceReads;
private final boolean logOutOfSequenceWrites;
private final Map> messageAnnotations;
private final Map> fieldAnnotations;
private final Map> enumAnnotations;
private Configuration(boolean logOutOfSequenceReads, boolean logOutOfSequenceWrites,
Map> messageAnnotations,
Map> fieldAnnotations,
Map> enumAnnotations) {
this.logOutOfSequenceReads = logOutOfSequenceReads;
this.logOutOfSequenceWrites = logOutOfSequenceWrites;
this.messageAnnotations = Collections.unmodifiableMap(messageAnnotations);
this.fieldAnnotations = Collections.unmodifiableMap(fieldAnnotations);
this.enumAnnotations = Collections.unmodifiableMap(enumAnnotations);
}
public boolean logOutOfSequenceReads() {
return logOutOfSequenceReads;
}
public boolean logOutOfSequenceWrites() {
return logOutOfSequenceWrites;
}
public Map> messageAnnotations() {
return messageAnnotations;
}
public Map> fieldAnnotations() {
return fieldAnnotations;
}
public Map> enumAnnotations() {
return enumAnnotations;
}
@Override
public String toString() {
return "Configuration{" +
"logOutOfSequenceReads=" + logOutOfSequenceReads +
", logOutOfSequenceWrites=" + logOutOfSequenceWrites +
", messageAnnotations=" + messageAnnotations +
", fieldAnnotations=" + fieldAnnotations +
", enumAnnotations=" + enumAnnotations +
'}';
}
public static final class Builder {
private boolean logOutOfSequenceReads = true;
private boolean logOutOfSequenceWrites = true;
private final Map> messageAnnotationBuilders = new HashMap>();
private final Map> fieldAnnotationBuilders = new HashMap>();
private final Map> enumAnnotationBuilders = new HashMap>();
public Builder() {
}
public boolean isLogOutOfSequenceReads() {
return logOutOfSequenceReads;
}
public Builder setLogOutOfSequenceReads(boolean logOutOfSequenceReads) {
this.logOutOfSequenceReads = logOutOfSequenceReads;
return this;
}
public boolean isLogOutOfSequenceWrites() {
return logOutOfSequenceWrites;
}
public Builder setLogOutOfSequenceWrites(boolean logOutOfSequenceWrites) {
this.logOutOfSequenceWrites = logOutOfSequenceWrites;
return this;
}
public AnnotationConfig.Builder messageAnnotation(String annotationName) {
AnnotationConfig.Builder builder = new AnnotationConfig.Builder(this, annotationName);
messageAnnotationBuilders.put(annotationName, builder);
return builder;
}
public AnnotationConfig.Builder enumAnnotation(String annotationName) {
AnnotationConfig.Builder builder = new AnnotationConfig.Builder(this, annotationName);
enumAnnotationBuilders.put(annotationName, builder);
return builder;
}
public AnnotationConfig.Builder fieldAnnotation(String annotationName) {
AnnotationConfig.Builder builder = new AnnotationConfig.Builder(this, annotationName);
fieldAnnotationBuilders.put(annotationName, builder);
return builder;
}
public Configuration build() {
messageAnnotation(TYPE_ID_ANNOTATION)
.attribute(AnnotationElement.Annotation.DEFAULT_ATTRIBUTE)
.intType()
.annotationMetadataCreator(new AnnotationMetadataCreator() {
@Override
public Integer create(Descriptor annotatedDescriptor, AnnotationElement.Annotation annotation) {
return (Integer) annotation.getDefaultAttributeValue().getValue();
}
});
enumAnnotation(TYPE_ID_ANNOTATION)
.attribute(AnnotationElement.Annotation.DEFAULT_ATTRIBUTE)
.intType()
.annotationMetadataCreator(new AnnotationMetadataCreator() {
@Override
public Integer create(EnumDescriptor annotatedDescriptor, AnnotationElement.Annotation annotation) {
return (Integer) annotation.getDefaultAttributeValue().getValue();
}
});
Map> messageAnnotations = new HashMap>(messageAnnotationBuilders.size());
for (AnnotationConfig.Builder annotationBuilder : messageAnnotationBuilders.values()) {
AnnotationConfig annotationConfig = annotationBuilder.buildAnnotationConfig();
messageAnnotations.put(annotationConfig.name(), annotationConfig);
}
Map> fieldAnnotations = new HashMap>(fieldAnnotationBuilders.size());
for (AnnotationConfig.Builder annotationBuilder : fieldAnnotationBuilders.values()) {
AnnotationConfig annotationConfig = annotationBuilder.buildAnnotationConfig();
fieldAnnotations.put(annotationConfig.name(), annotationConfig);
}
Map> enumAnnotations = new HashMap>(enumAnnotationBuilders.size());
for (AnnotationConfig.Builder annotationBuilder : enumAnnotationBuilders.values()) {
AnnotationConfig annotationConfig = annotationBuilder.buildAnnotationConfig();
enumAnnotations.put(annotationConfig.name(), annotationConfig);
}
return new Configuration(logOutOfSequenceReads, logOutOfSequenceWrites, messageAnnotations, fieldAnnotations, enumAnnotations);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy