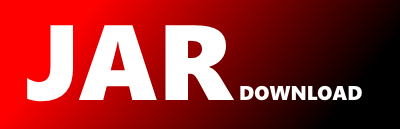
org.infinispan.server.resp.Resp3Handler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-server-resp Show documentation
Show all versions of infinispan-server-resp Show documentation
Infinispan Resp Protocol Server
package org.infinispan.server.resp;
import static org.infinispan.server.resp.serialization.RespConstants.CRLF_STRING;
import java.util.List;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.ScheduledExecutorService;
import org.infinispan.AdvancedCache;
import org.infinispan.Cache;
import org.infinispan.commons.dataconversion.MediaType;
import org.infinispan.context.Flag;
import org.infinispan.factories.GlobalComponentRegistry;
import org.infinispan.factories.KnownComponentNames;
import org.infinispan.multimap.impl.EmbeddedMultimapListCache;
import org.infinispan.multimap.impl.EmbeddedMultimapPairCache;
import org.infinispan.multimap.impl.EmbeddedMultimapSortedSetCache;
import org.infinispan.multimap.impl.EmbeddedSetCache;
import org.infinispan.security.AuthorizationManager;
import org.infinispan.security.AuthorizationPermission;
import org.infinispan.security.actions.SecurityActions;
import org.infinispan.server.resp.commands.Resp3Command;
import org.infinispan.util.concurrent.BlockingManager;
import io.netty.channel.ChannelHandlerContext;
public class Resp3Handler extends Resp3AuthHandler {
private static final byte[] CRLF_BYTES = CRLF_STRING.getBytes();
protected AdvancedCache ignorePreviousValueCache;
protected EmbeddedMultimapListCache listMultimap;
protected EmbeddedMultimapPairCache mapMultimap;
protected EmbeddedSetCache embeddedSetCache;
protected EmbeddedMultimapSortedSetCache sortedSetMultimap;
protected final ScheduledExecutorService scheduler;
protected final BlockingManager blockingManager;
private final MediaType valueMediaType;
Resp3Handler(RespServer respServer, MediaType valueMediaType) {
super(respServer);
this.valueMediaType = valueMediaType;
GlobalComponentRegistry gcr = SecurityActions.getGlobalComponentRegistry(cache.getCacheManager());
this.scheduler = gcr.getComponent(ScheduledExecutorService.class, KnownComponentNames.TIMEOUT_SCHEDULE_EXECUTOR);
this.blockingManager = gcr.getComponent(BlockingManager.class);
}
@Override
public void setCache(AdvancedCache cache) {
super.setCache(cache);
ignorePreviousValueCache = cache.withFlags(Flag.SKIP_CACHE_LOAD, Flag.IGNORE_RETURN_VALUES);
Cache toMultimap = cache.withMediaType(MediaType.APPLICATION_OCTET_STREAM, valueMediaType);
listMultimap = new EmbeddedMultimapListCache<>(toMultimap);
mapMultimap = new EmbeddedMultimapPairCache<>(toMultimap);
embeddedSetCache = new EmbeddedSetCache<>(toMultimap);
sortedSetMultimap = new EmbeddedMultimapSortedSetCache<>(toMultimap);
}
public EmbeddedMultimapListCache getListMultimap() {
return listMultimap;
}
public EmbeddedMultimapPairCache getHashMapMultimap() {
return mapMultimap;
}
public EmbeddedSetCache getEmbeddedSetCache() {
return embeddedSetCache;
}
public EmbeddedMultimapSortedSetCache getSortedSeMultimap() {
return sortedSetMultimap;
}
public ScheduledExecutorService getScheduler() {
return scheduler;
}
public BlockingManager getBlockingManager() {
return blockingManager;
}
@Override
protected CompletionStage actualHandleRequest(ChannelHandlerContext ctx, RespCommand type,
List arguments) {
if (type instanceof Resp3Command) {
Resp3Command resp3Command = (Resp3Command) type;
return resp3Command.perform(this, ctx, arguments);
}
return super.actualHandleRequest(ctx, type, arguments);
}
public AdvancedCache ignorePreviousValuesCache() {
return ignorePreviousValueCache;
}
public CompletionStage delegate(ChannelHandlerContext ctx,
RespCommand command,
List arguments) {
return super.actualHandleRequest(ctx, command, arguments);
}
public void checkPermission(AuthorizationPermission authorizationPermission) {
AuthorizationManager authorizationManager = cache.getAuthorizationManager();
if (authorizationManager != null) {
authorizationManager.checkPermission(authorizationPermission);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy