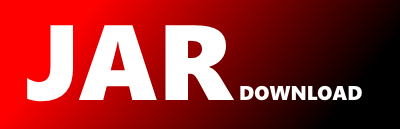
org.infinispan.server.resp.commands.generic.EXPIRE Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-server-resp Show documentation
Show all versions of infinispan-server-resp Show documentation
Infinispan Resp Protocol Server
package org.infinispan.server.resp.commands.generic;
import static org.infinispan.server.resp.Util.fromUnixTime;
import static org.infinispan.server.resp.Util.toUnixTime;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.TimeUnit;
import org.infinispan.AdvancedCache;
import org.infinispan.commons.dataconversion.MediaType;
import org.infinispan.server.resp.Resp3Handler;
import org.infinispan.server.resp.RespCommand;
import org.infinispan.server.resp.RespRequestHandler;
import org.infinispan.server.resp.commands.ArgumentUtils;
import org.infinispan.server.resp.commands.Resp3Command;
import org.infinispan.server.resp.serialization.Resp3Response;
import io.netty.channel.ChannelHandlerContext;
/**
* EXPIRE Resp Command
*
* @link EXPIRE
* @since 15.0
*/
public class EXPIRE extends RespCommand implements Resp3Command {
public static final CompletableFuture NOT_APPLIED = CompletableFuture.completedFuture(0L);
public static final CompletableFuture APPLIED = CompletableFuture.completedFuture(1L);
enum Mode {
NONE, NX, XX, GT, LT
}
private final boolean unixTime;
private final boolean seconds;
public EXPIRE() {
this(false, true);
}
protected EXPIRE(boolean at, boolean seconds) {
super(-3, 1, 1, 1);
this.unixTime = at;
this.seconds = seconds;
}
@Override
public CompletionStage perform(Resp3Handler handler,
ChannelHandlerContext ctx,
List arguments) {
byte[] key = arguments.get(0);
long expiration = ArgumentUtils.toLong(arguments.get(1));
if (seconds) {
expiration = TimeUnit.SECONDS.toMillis(expiration);
}
Mode mode = Mode.NONE;
if (arguments.size() == 3) {
// Handle mode
mode = Mode.valueOf(new String(arguments.get(2), StandardCharsets.US_ASCII).toUpperCase());
}
return handler.stageToReturn(expire(handler, key, expiration, mode, unixTime), ctx, Resp3Response.INTEGER);
}
private static CompletionStage expire(Resp3Handler handler, byte[] key, long expiration, Mode mode, boolean unixTime) {
MediaType vmt = handler.cache().getValueDataConversion().getStorageMediaType();
final AdvancedCache acm = handler.cache().withMediaType(MediaType.APPLICATION_OCTET_STREAM, vmt);
return acm.getCacheEntryAsync(key).thenCompose(e -> {
if (e == null) {
return NOT_APPLIED;
} else {
long ttl = e.getLifespan();
if (unixTime) {
ttl = toUnixTime(ttl, handler.respServer().getTimeService());
} else if (ttl >= 0) {
ttl = e.getLifespan();
}
switch (mode) {
case NX:
if (ttl >= 0) {
return NOT_APPLIED;
}
break;
case XX:
if (ttl < 0) {
return NOT_APPLIED;
}
break;
case GT:
if (expiration < ttl) {
return NOT_APPLIED;
}
break;
case LT:
if (expiration > ttl) {
return NOT_APPLIED;
}
break;
}
CompletableFuture replace;
if (unixTime) {
replace = acm.replaceAsync(e.getKey(), e.getValue(), e.getValue(), fromUnixTime(expiration, handler.respServer().getTimeService()), TimeUnit.MILLISECONDS);
} else {
replace = acm.replaceAsync(e.getKey(), e.getValue(), e.getValue(), expiration, TimeUnit.MILLISECONDS);
}
return replace.thenCompose(b -> {
if (b) {
return APPLIED;
} else {
return expire(handler, key, expiration, mode, unixTime);
}
});
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy