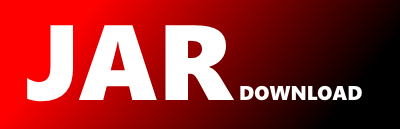
org.infinispan.server.resp.commands.hash.HINCRBYFLOAT Maven / Gradle / Ivy
Show all versions of infinispan-server-resp Show documentation
package org.infinispan.server.resp.commands.hash;
import java.util.List;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.atomic.AtomicInteger;
import org.infinispan.multimap.impl.EmbeddedMultimapPairCache;
import org.infinispan.server.resp.Resp3Handler;
import org.infinispan.server.resp.RespCommand;
import org.infinispan.server.resp.RespErrorUtil;
import org.infinispan.server.resp.RespRequestHandler;
import org.infinispan.server.resp.commands.ArgumentUtils;
import org.infinispan.server.resp.commands.Resp3Command;
import org.infinispan.server.resp.serialization.Resp3Response;
import io.netty.channel.ChannelHandlerContext;
/**
* `HINCRBYFLOAT key field increment
` command.
*
* Increments the value at the field
in the hash map stored at key
by increment
.
* The command fails if the stored value is not a number.
*
* @since 15.0
* @see Redis Documentation
* @author José Bolina
*/
public class HINCRBYFLOAT extends RespCommand implements Resp3Command {
private static final int SUCCESS = 0;
private static final int NOT_A_FLOAT = 1;
private static final int NAN_OR_INF = 2;
public HINCRBYFLOAT() {
super(4, 1, 1, 1);
}
@Override
public CompletionStage perform(Resp3Handler handler, ChannelHandlerContext ctx, List arguments) {
EmbeddedMultimapPairCache multimap = handler.getHashMapMultimap();
double delta = ArgumentUtils.toDouble(arguments.get(2));
if (!ArgumentUtils.isFloatValid(delta)) {
RespErrorUtil.nanOrInfinity(handler.allocator());
return handler.myStage();
}
AtomicInteger status = new AtomicInteger(SUCCESS);
CompletionStage cs = multimap.compute(arguments.get(0), arguments.get(1), (ignore, prev) -> {
if (prev == null) return arguments.get(2);
try {
double prevDouble = ArgumentUtils.toDouble(prev);
double after = prevDouble + delta;
if (!ArgumentUtils.isFloatValid(after)) {
status.set(NAN_OR_INF);
return prev;
}
return ArgumentUtils.toByteArray(prevDouble + delta);
} catch (NumberFormatException nfe) {
status.set(NOT_A_FLOAT);
return prev;
}
});
return handler.stageToReturn(cs, ctx, (res, alloc) -> {
switch (status.get()) {
case NOT_A_FLOAT -> RespErrorUtil.customError("hash value is not a float", alloc);
case NAN_OR_INF -> RespErrorUtil.nanOrInfinity(alloc);
// Yes, the double value return as a bulk string...
default -> Resp3Response.string(res, alloc);
}
});
}
}