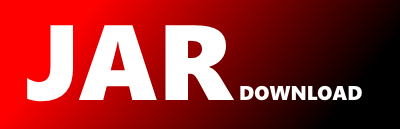
org.infinispan.server.resp.commands.iteration.BaseIterationCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-server-resp Show documentation
Show all versions of infinispan-server-resp Show documentation
Infinispan Resp Protocol Server
package org.infinispan.server.resp.commands.iteration;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.CompletionStage;
import org.infinispan.AdvancedCache;
import org.infinispan.commons.dataconversion.MediaType;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.reactive.publisher.impl.DeliveryGuarantee;
import org.infinispan.server.iteration.IterableIterationResult;
import org.infinispan.server.iteration.IterationInitializationContext;
import org.infinispan.server.iteration.IterationManager;
import org.infinispan.server.iteration.IterationState;
import org.infinispan.server.resp.Resp3Handler;
import org.infinispan.server.resp.RespCommand;
import org.infinispan.server.resp.RespRequestHandler;
import org.infinispan.server.resp.commands.Resp3Command;
import org.infinispan.server.resp.serialization.ByteBufferUtils;
import org.infinispan.server.resp.serialization.Resp3Response;
import org.infinispan.server.resp.serialization.Resp3Type;
import org.infinispan.server.resp.serialization.RespConstants;
import org.infinispan.util.concurrent.BlockingManager;
import io.netty.channel.ChannelHandlerContext;
public abstract class BaseIterationCommand extends RespCommand implements Resp3Command {
private static final String INITIAL_CURSOR = "0";
protected BaseIterationCommand(int arity, int firstKeyPos, int lastKeyPos, int steps) {
super(arity, firstKeyPos, lastKeyPos, steps);
}
protected byte[] getMatch(List arguments) {
return null;
}
@Override
public final CompletionStage perform(Resp3Handler handler, ChannelHandlerContext ctx, List arguments) {
IterationArguments args = IterationArguments.parse(handler, arguments, getMatch(arguments));
if (args == null) return handler.myStage();
IterationManager manager = retrieveIterationManager(handler);
String cursor = cursor(arguments);
if (INITIAL_CURSOR.equals(cursor)) {
CompletionStage initialization = initializeIteration(handler, arguments);
if (initialization != null) {
return handler.stageToReturn(initialization.thenCompose(iic -> initializeAndIterate(handler, ctx, manager, args, iic)), ctx);
}
return initializeAndIterate(handler, ctx, manager, args, null);
}
return iterate(handler, ctx, manager, cursor, args);
}
private CompletionStage initializeAndIterate(Resp3Handler handler, ChannelHandlerContext ctx,
IterationManager manager, IterationArguments arguments,
IterationInitializationContext iic) {
AdvancedCache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy