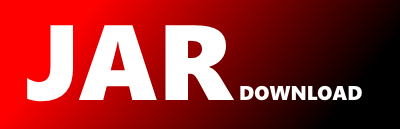
org.infinispan.server.resp.commands.string.GETEX Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-server-resp Show documentation
Show all versions of infinispan-server-resp Show documentation
Infinispan Resp Protocol Server
package org.infinispan.server.resp.commands.string;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.TimeUnit;
import org.infinispan.AdvancedCache;
import org.infinispan.Cache;
import org.infinispan.commons.time.TimeService;
import org.infinispan.commons.util.concurrent.CompletableFutures;
import org.infinispan.server.resp.Resp3Handler;
import org.infinispan.server.resp.RespCommand;
import org.infinispan.server.resp.RespRequestHandler;
import org.infinispan.server.resp.commands.Resp3Command;
import org.infinispan.server.resp.operation.GetexOperation;
import org.infinispan.server.resp.serialization.Resp3Response;
import io.netty.channel.ChannelHandlerContext;
/**
* GETEX Resp Command
*
* @link https://redis.io/commands/getex/
* @since 15.0
*/
public class GETEX extends RespCommand implements Resp3Command {
public GETEX() {
super(-2, 1, 1, 1);
}
@Override
public CompletionStage perform(Resp3Handler handler,
ChannelHandlerContext ctx,
List arguments) {
if (arguments.size() > 1) {
return handler
.stageToReturn(performOperation(handler.cache(), arguments, handler.respServer().getTimeService()), ctx,
Resp3Response.BULK_STRING_BYTES);
}
byte[] keyBytes = arguments.get(0);
return handler.stageToReturn(handler.cache().getAsync(keyBytes), ctx, Resp3Response.BULK_STRING_BYTES);
}
private static CompletionStage performOperation(AdvancedCache cache,
List arguments, TimeService timeService) {
long expirationMs = GetexOperation.parseExpiration(arguments, timeService);
return getex(cache, arguments.get(0), expirationMs);
}
static CompletionStage getex(Cache cache, byte[] key, long expirationMs) {
return cache.getAsync(key)
.thenCompose(currentValueBytes -> {
if (currentValueBytes == null) {
return CompletableFutures.completedNull();
}
return cache.replaceAsync(key, currentValueBytes, currentValueBytes, expirationMs, TimeUnit.MILLISECONDS)
.thenCompose(replaced -> {
if (replaced) {
return CompletableFuture.completedFuture(currentValueBytes);
}
return getex(cache, key, expirationMs);
});
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy