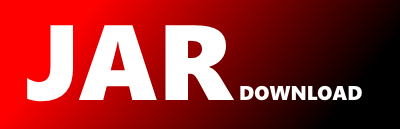
org.infinispan.server.resp.meta.ClientMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-server-resp Show documentation
Show all versions of infinispan-server-resp Show documentation
Infinispan Resp Protocol Server
package org.infinispan.server.resp.meta;
import static java.util.concurrent.atomic.AtomicLongFieldUpdater.newUpdater;
import java.util.concurrent.atomic.AtomicLongFieldUpdater;
/**
* Metadata for `INFO
` command.
*
* Holds metadata about client connections. All the values are specific to a single node.
*
*
* @since 15.1
* @see org.infinispan.server.resp.commands.INFO
*/
public final class ClientMetadata {
private static final AtomicLongFieldUpdater CONNECTED = newUpdater(ClientMetadata.class, "connectedClients");
private static final AtomicLongFieldUpdater BLOCKED = newUpdater(ClientMetadata.class, "blockedClients");
private static final AtomicLongFieldUpdater PUB_SUB = newUpdater(ClientMetadata.class, "pubSubClients");
private static final AtomicLongFieldUpdater WATCH = newUpdater(ClientMetadata.class, "watchingClients");
private static final AtomicLongFieldUpdater KEYS_BLOCKED = newUpdater(ClientMetadata.class, "blockedKeys");
private static final AtomicLongFieldUpdater KEYS_WATCH = newUpdater(ClientMetadata.class, "watchedKeys");
/**
* Number of client connections
*/
private volatile long connectedClients = 0;
/**
* Number of clients pending on a blocking call (BLPOP, BRPOP, BRPOPLPUSH, BLMOVE, BZPOPMIN, BZPOPMAX)
*/
private volatile long blockedClients = 0;
/**
* Number of clients in pubsub mode (SUBSCRIBE, PSUBSCRIBE, SSUBSCRIBE)
*/
private volatile long pubSubClients = 0;
/**
* Number of clients in watching mode (WATCH)
*/
private volatile long watchingClients = 0;
/**
* Number of blocking keys
*/
private volatile long blockedKeys = 0;
/**
* Number of watched keys
*/
private volatile long watchedKeys = 0;
public void incrementConnectedClients() {
CONNECTED.incrementAndGet(this);
}
public void decrementConnectedClients() {
CONNECTED.decrementAndGet(this);
}
public void incrementBlockedClients() {
BLOCKED.incrementAndGet(this);
}
public void decrementBlockedClients() {
BLOCKED.decrementAndGet(this);
}
public void incrementPubSubClients() {
PUB_SUB.incrementAndGet(this);
}
public void decrementPubSubClients() {
PUB_SUB.decrementAndGet(this);
}
public void incrementWatchingClients() {
WATCH.incrementAndGet(this);
}
public void decrementWatchingClients() {
WATCH.decrementAndGet(this);
}
public long getConnectedClients() {
return CONNECTED.get(this);
}
public long getBlockedClients() {
return BLOCKED.get(this);
}
public long getPubSubClients() {
return PUB_SUB.get(this);
}
public long getWatchingClients() {
return WATCH.get(this);
}
public void recordBlockedKeys(long value) {
KEYS_BLOCKED.addAndGet(this, value);
}
public void recordWatchedKeys(long value) {
KEYS_WATCH.addAndGet(this, value);
}
public long getBlockedKeys() {
return KEYS_BLOCKED.get(this);
}
public long getWatchedKeys() {
return KEYS_WATCH.get(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy