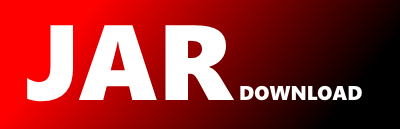
org.infinispan.server.resp.serialization.CollectionSerializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-server-resp Show documentation
Show all versions of infinispan-server-resp Show documentation
Infinispan Resp Protocol Server
package org.infinispan.server.resp.serialization;
import java.util.Collection;
import java.util.Set;
import org.infinispan.server.resp.ByteBufPool;
/**
* Serialize collection types into the RESP3 equivalent.
*
* @since 15.0
* @author José Bolina
*/
final class CollectionSerializer {
/**
* Abstract the serialization of collections.
*
* @param objects: The values to serialize.
* @param alloc: The buffer allocator.
* @param symbol: The prefix symbol that represents the collection type.
*/
private static void serialize(Collection> objects, ByteBufPool alloc, byte symbol, SerializationHint.SimpleHint hint) {
// First, writes the prefix.
// RESP: \r\n
ByteBufferUtils.writeNumericPrefix(symbol, objects.size(), alloc);
// Write each element individually.
// The values are heterogeneous, they can be primitives or another aggregate type.
for (Object object : objects) {
hint.serialize(object, alloc);
}
}
/**
* Represent an ordered aggregate of elements.
*
*
* The prefix is an asterisk ({@link RespConstants#ARRAY}), followed by the base-10 representation of the number of elements
* in the list and the terminator. The collection is heterogeneous and contains varying types of values. Therefore, each
* collection element is serialized individually, following the correct representation.
*
*/
static final class ArraySerializer implements NestedResponseSerializer, SerializationHint.SimpleHint> {
static final ArraySerializer INSTANCE = new ArraySerializer();
@Override
public void accept(Collection> objects, ByteBufPool alloc, SerializationHint.SimpleHint hint) {
// RESP: *\r\n...
serialize(objects, alloc, RespConstants.ARRAY, hint);
}
@Override
public boolean test(Object object) {
// Handle any collection which is not a set.
return object instanceof Collection> && !(object instanceof Set>);
}
}
/**
* Represent an unordered aggregate of unique elements.
*
*
* Sets behave like {@link ArraySerializer}, but the elements do not have an order, and the collection contains strictly
* unique elements. The prefix for a set is the tilde symbol ({@link RespConstants#SET}).
*
*/
static final class SetSerializer implements NestedResponseSerializer, SerializationHint.SimpleHint> {
static final SetSerializer INSTANCE = new SetSerializer();
@Override
public void accept(Set> objects, ByteBufPool alloc, SerializationHint.SimpleHint hint) {
// RESP: ~\r\n...
serialize(objects, alloc, RespConstants.SET, hint);
}
@Override
public boolean test(Object object) {
// Accept any instance of sets.
return object instanceof Set>;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy