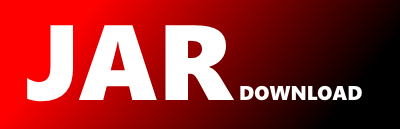
org.infinispan.server.tasks.DistributedServerTask Maven / Gradle / Ivy
package org.infinispan.server.tasks;
import java.util.function.Function;
import org.infinispan.Cache;
import org.infinispan.commons.CacheException;
import org.infinispan.commons.dataconversion.MediaType;
import org.infinispan.commons.marshall.Marshaller;
import org.infinispan.commons.marshall.ProtoStreamTypeIds;
import org.infinispan.commons.marshall.StreamingMarshaller;
import org.infinispan.factories.GlobalComponentRegistry;
import org.infinispan.manager.EmbeddedCacheManager;
import org.infinispan.protostream.annotations.ProtoFactory;
import org.infinispan.protostream.annotations.ProtoField;
import org.infinispan.protostream.annotations.ProtoTypeId;
import org.infinispan.tasks.TaskContext;
/**
* @author Michal Szynkiewicz, [email protected]
*/
@ProtoTypeId(ProtoStreamTypeIds.DISTRIBUTED_SERVER_TASK)
public class DistributedServerTask implements Function {
@ProtoField(1)
final String taskName;
@ProtoField(2)
final String cacheName;
@ProtoField(3)
final TaskContext context;
@ProtoFactory
public DistributedServerTask(String taskName, String cacheName, TaskContext context) {
this.cacheName = cacheName;
this.taskName = taskName;
this.context = context;
}
@Override
public T apply(EmbeddedCacheManager embeddedCacheManager) {
Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy