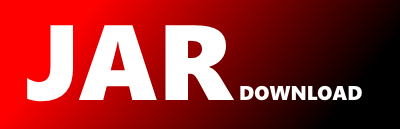
org.influxdb.dto.QueryResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of influxdb-java Show documentation
Show all versions of influxdb-java Show documentation
Java API to access the InfluxDB REST API
The newest version!
package org.influxdb.dto;
import java.util.List;
import java.util.Map;
/**
* {Purpose of This Type}.
*
* {Other Notes Relating to This Type (Optional)}
*
* @author stefan
*
*/
// {
// "results": [
// {
// "series": [{}],
// "error": "...."
// }
// ],
// "error": "...."
// }
// {"results":[{"series":[{"name":"cpu","columns":["time","value"],
// "values":[["2015-06-06T14:55:27.195Z",90],["2015-06-06T14:56:24.556Z",90]]}]}]}
// {"results":[{"series":[{"name":"databases","columns":["name"],"values":[["mydb"]]}]}]}
public class QueryResult {
private List results;
private String error;
/**
* @return the results
*/
public List getResults() {
return this.results;
}
/**
* @param results
* the results to set
*/
public void setResults(final List results) {
this.results = results;
}
/**
* Checks if this QueryResult has an error message.
*
* @return true
if there is an error message, false
if not.
*/
public boolean hasError() {
return this.error != null;
}
/**
* @return the error
*/
public String getError() {
return this.error;
}
/**
* @param error
* the error to set
*/
public void setError(final String error) {
this.error = error;
}
public static class Result {
private List series;
private String error;
/**
* @return the series
*/
public List getSeries() {
return this.series;
}
/**
* @param series
* the series to set
*/
public void setSeries(final List series) {
this.series = series;
}
/**
* Checks if this Result has an error message.
*
* @return true
if there is an error message,
* false
if not.
*/
public boolean hasError() {
return this.error != null;
}
/**
* @return the error
*/
public String getError() {
return this.error;
}
/**
* @param error
* the error to set
*/
public void setError(final String error) {
this.error = error;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("Result [series=");
builder.append(this.series);
builder.append(", error=");
builder.append(this.error);
builder.append("]");
return builder.toString();
}
}
public static class Series {
private String name;
private Map tags;
private List columns;
private List> values;
/**
* @return the name
*/
public String getName() {
return this.name;
}
/**
* @param name
* the name to set
*/
public void setName(final String name) {
this.name = name;
}
/**
* @return the tags
*/
public Map getTags() {
return this.tags;
}
/**
* @param tags
* the tags to set
*/
public void setTags(final Map tags) {
this.tags = tags;
}
/**
* @return the columns
*/
public List getColumns() {
return this.columns;
}
/**
* @param columns
* the columns to set
*/
public void setColumns(final List columns) {
this.columns = columns;
}
/**
* @return the values
*/
public List> getValues() {
return this.values;
}
/**
* @param values
* the values to set
*/
public void setValues(final List> values) {
this.values = values;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("Series [name=");
builder.append(this.name);
builder.append(", tags=");
builder.append(this.tags);
builder.append(", columns=");
builder.append(this.columns);
builder.append(", values=");
builder.append(this.values);
builder.append("]");
return builder.toString();
}
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("QueryResult [results=");
builder.append(this.results);
builder.append(", error=");
builder.append(this.error);
builder.append("]");
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy