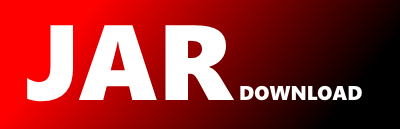
org.influxdb.impl.InfluxDBMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of influxdb-java Show documentation
Show all versions of influxdb-java Show documentation
Java API to access the InfluxDB REST API
The newest version!
package org.influxdb.impl;
import org.influxdb.InfluxDB;
import org.influxdb.annotation.Measurement;
import org.influxdb.dto.Point;
import org.influxdb.dto.Query;
import org.influxdb.dto.QueryResult;
import java.util.List;
public class InfluxDBMapper extends InfluxDBResultMapper {
private final InfluxDB influxDB;
public InfluxDBMapper(final InfluxDB influxDB) {
this.influxDB = influxDB;
}
public List query(final Query query, final Class clazz, final String measurementName) {
QueryResult queryResult = influxDB.query(query);
return toPOJO(queryResult, clazz, measurementName);
}
public List query(final Query query, final Class clazz) {
throwExceptionIfMissingAnnotation(clazz);
QueryResult queryResult = influxDB.query(query);
return toPOJO(queryResult, clazz);
}
public List query(final Class clazz) {
throwExceptionIfMissingAnnotation(clazz);
String measurement = getMeasurementName(clazz);
String database = getDatabaseName(clazz);
if ("[unassigned]".equals(database)) {
throw new IllegalArgumentException(
Measurement.class.getSimpleName()
+ " of class "
+ clazz.getName()
+ " should specify a database value for this operation");
}
QueryResult queryResult = influxDB.query(new Query("SELECT * FROM " + measurement, database));
return toPOJO(queryResult, clazz);
}
public void save(final T model) {
throwExceptionIfMissingAnnotation(model.getClass());
Class> modelType = model.getClass();
String database = getDatabaseName(modelType);
String retentionPolicy = getRetentionPolicy(modelType);
Point.Builder pointBuilder = Point.measurementByPOJO(modelType).addFieldsFromPOJO(model);
Point point = pointBuilder.build();
if ("[unassigned]".equals(database)) {
influxDB.write(point);
} else {
influxDB.write(database, retentionPolicy, point);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy