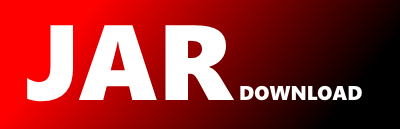
com.i2soft.util.StringMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2up-java-sdk Show documentation
Show all versions of i2up-java-sdk Show documentation
Information2 United Data Management Platform SDK for Java
package com.i2soft.util;
import okhttp3.MediaType;
import okhttp3.RequestBody;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.*;
/**
* 封装 http 请求参数,query/body 的参数转换(json/url args)
* put:key: String, value: String | Integer | Long | String[] | Integer[] | Long[] | StringMap | Map
*/
public final class StringMap {
private Map map;
public StringMap() {
this(new HashMap());
}
public StringMap(Map map) {
if (map != null && map.size() > 0) {
this.map = (Map) map;
} else {
this.map = new HashMap<>();
}
}
// put
public StringMap put(String key, String value) {
map.put(key, value);
return this;
}
public StringMap put(String key, Integer value) {
map.put(key, value);
return this;
}
public StringMap put(String key, Long value) {
map.put(key, value);
return this;
}
public StringMap put(String key, Double value) {
map.put(key, value);
return this;
}
// put arr
public StringMap put(String key, String[] value) {
map.put(key, value);
return this;
}
public StringMap put(String key, Integer[] value) {
map.put(key, value);
return this;
}
public StringMap put(String key, Long[] value) {
map.put(key, value);
return this;
}
// put map
public StringMap put(String key, StringMap value) {
map.put(key, value.map());
return this;
}
public StringMap put(String key, Map value) {
map.put(key, value);
return this;
}
// putNotNull
public StringMap putNotEmpty(String key, String value) {
if (!StringUtils.isNullOrEmpty(value)) {
map.put(key, value);
}
return this;
}
public StringMap putNotNull(String key, Integer value) {
if (value != null) {
map.put(key, value);
}
return this;
}
public StringMap putNotNull(String key, Long value) {
if (value != null) {
map.put(key, value);
}
return this;
}
// putNotNull arr
public StringMap putNotEmpty(String key, String[] value) {
if (value != null && value.length > 0) {
map.put(key, value);
}
return this;
}
public StringMap putNotEmpty(String key, Integer[] value) {
if (value != null && value.length > 0) {
map.put(key, value);
}
return this;
}
public StringMap putNotEmpty(String key, Long[] value) {
if (value != null && value.length > 0) {
map.put(key, value);
}
return this;
}
public StringMap putNotEmpty(String key, StringMap[] value) {
if (value != null && value.length > 0) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy