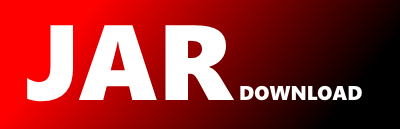
com.i2soft.active.v20220622.Kafka Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2up-java-sdk Show documentation
Show all versions of i2up-java-sdk Show documentation
Information2 United Data Management Platform SDK for Java
The newest version!
package com.i2soft.active.v20220622;
import com.i2soft.common.Auth;
import com.i2soft.http.I2Rs;
import com.i2soft.http.I2softException;
import com.i2soft.http.Response;
import com.i2soft.util.StringMap;
import java.util.Map;
public final class Kafka extends Rule {
/**
* Auth 对象
*/
private final Auth auth;
/**
* 构建一个新对象
*
* @param auth Auth对象
*/
public Kafka(Auth auth) {
super(auth);
this.auth = auth;
}
/**
* 异构-新建
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs createHeteroRule(StringMap args) throws I2softException {
String url = String.format("%s/hetero/rule", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-删除
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs deleteHeteroRule(StringMap args) throws I2softException {
String url = String.format("%s/hetero", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-获取列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listHeteroRule(StringMap args) throws I2softException {
String url = String.format("%s/hetero/rule", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 异构-查看topic
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createHeteroTopic(StringMap args) throws I2softException {
String url = String.format("%s/hetero/topic", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 异构-查看消费者
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createConsumer(StringMap args) throws I2softException {
String url = String.format("%s/hetero/view_consumer", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 异构-消费
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs consumer(StringMap args) throws I2softException {
String url = String.format("%s/hetero/consumer", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-消费-新建
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2CreateRs createConsumerRule(StringMap args) throws I2softException {
String url = String.format("%s/hetero/consumer/rule", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 异构-消费-修改
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs modifyConsumerRule(StringMap args) throws I2softException {
String url = String.format("%s/hetero/consumer/rule", auth.cc_url);
Response r = auth.client.put(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-消费-删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteConsumerRules(StringMap args) throws I2softException {
String url = String.format("%s/hetero/consumer/rule", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 异构-消费-状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listConsumerStatus(StringMap args) throws I2softException {
String url = String.format("%s/hetero/consumer/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 异构-消费规则-操作base
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs operateRule(StringMap args) throws I2softException {
String url = String.format("%s/hetero/consumer/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-消费规则 - 操作base
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs operateRule(String uuid, StringMap args) throws I2softException {
args.put("uuid", uuid);
return this.operateRule(args);
}
/**
* 异构-消费规则 - 操作 - 停止
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopConsumerRule(String uuid, StringMap args) throws I2softException {
args.put("operate", STOP);
return operateRule(uuid, args);
}
/**
* 异构-消费规则 - 操作 - 继续
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs resumeConsumerRule(String uuid, StringMap args) throws I2softException {
args.put("operate", RESUME);
return operateRule(uuid, args);
}
/**
* 异构-消费规则 - 操作 - 重启
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs restartConsumerRule(String uuid, StringMap args) throws I2softException {
args.put("operate", RESTART);
return operateRule(uuid, args);
}
/**
* 异构-消费-获取规则列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listConsumerRules(StringMap args) throws I2softException {
String url = String.format("%s/hetero/consumer/viewtype", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 异构-消费-获取单条规则
*
* @param uuid: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeConsumerRule(String uuid) throws I2softException {
String url = String.format("%s/hetero/consumer/rule/%s", auth.cc_url, uuid);
Response r = auth.client.get(url);
return r.jsonToMap();
}
/**
* 异构-拓扑-新建
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs createHeteroGraph(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-拓扑-添加
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs addHeteroGraph(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph/add", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-拓扑-获取列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listHeteroGraph(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph/list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 异构-拓扑-运行拓扑
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs runHeteroGraph(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph/run", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-拓扑-停止拓扑
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopHeteroGraph(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph/stop", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-拓扑-状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listGraphStatus(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 异构-拓扑-删除拓扑
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs deleteHeteroGraph(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 异构-拓扑-拓扑详情
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map descriptGraphDetail(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph/detail", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 异构-拓扑图-获取拓扑图
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listGraph(StringMap args) throws I2softException {
String url = String.format("%s/hetero/graph/graph", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy