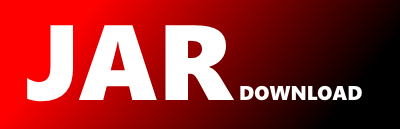
com.i2soft.active.v20240311.OracleRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2up-java-sdk Show documentation
Show all versions of i2up-java-sdk Show documentation
Information2 United Data Management Platform SDK for Java
The newest version!
package com.i2soft.active.v20240311;
import com.i2soft.http.I2Rs;
import com.i2soft.http.I2softException;
import com.i2soft.http.Response;
import com.i2soft.common.Auth;
import com.i2soft.util.StringMap;
import java.util.Map;
public final class OracleRule {
/**
* Auth 对象
*/
private final Auth auth;
/**
* 构建一个新对象
*
* @param auth Auth对象
*/
public OracleRule(Auth auth) {
this.auth = auth;
}
/**
* 同步规则 - 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listSyncRules(StringMap args) throws I2softException {
String url = String.format("%s/active/rule", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public I2Rs.I2CreateRs createOracleRule(StringMap args) throws I2softException {
String url = String.format("%s/active/rule", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 同步规则 - 批量新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createBatchOracleRule(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/batch", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 修改
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public I2Rs.I2CreateRs modifyOracleRule(StringMap args) throws I2softException {
String url = String.format("%s/active/rule", auth.cc_url);
Response r = auth.client.put(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 同步规则 - 批量修改
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyOracleRuleBatch(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/batch", auth.cc_url);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteOracleRule(StringMap args) throws I2softException {
String url = String.format("%s/active/rule", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 同步规则-数据库预检(已废弃)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeRuleDbCheckMult(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/db_check_single", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则-获取单个
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRules(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/active/rule/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map operateOracleRule(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map resumeOracleRule(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map stopOracleRule(StringMap args) throws I2softException {
args.put("operate", "stop");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map restartOracleRule(StringMap args) throws I2softException {
args.put("operate", "restart");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map stopAndStopanalysisOracleRule(StringMap args) throws I2softException {
args.put("operate", "stop_and_stopanalysis");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map stopAnalysisOracleRule(StringMap args) throws I2softException {
args.put("operate", "stop_analysis");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map startAnalysisOracleRule(StringMap args) throws I2softException {
args.put("operate", "start_analysis");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map restartAnalysisOracleRule(StringMap args) throws I2softException {
args.put("operate", "restart_analysis");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map startScheduleOracleRule(StringMap args) throws I2softException {
args.put("operate", "start_schedule");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map stopScheduleOracleRule(StringMap args) throws I2softException {
args.put("operate", "stop_schedule");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map duplicateOracleRule(StringMap args) throws I2softException {
args.put("operate", "duplicate");
String url = String.format("%s/active/rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listSyncRulesStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 同步规则-表修复
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs describeRuleTableFix(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/table_fix", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 同步规则-获取scn号
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeRuleGetScn(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/get_scn", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 同步规则-偏移量信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listKafkaOffsetInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/kafka_offset", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 表比较 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public I2Rs.I2CreateRs createTbCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 表比较 - 获取单个
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeTbCmp(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 表比较 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteTbCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 表比较 - 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listTbCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 表比较/数据检查 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listTbCmpStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/status", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则-日志
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listRuleLog(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/log", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 表比较-操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopTbCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 表比较 - 历史结果(查看表比较时间结果集)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listTbCmpResultTimeList(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/result_time_list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 表比较-比较结果的删除
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs describeTbCmpResuluTimeList(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/result_time_list", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 表比较-比较任务结果
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeTbCmpResult(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/result", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 表比较-错误信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeTbCmpErrorMsg(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/error_msg", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 表比较-表比对的详细信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeTbCmpCmpDesc(StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/cmp_describe", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 表比较-比较结果
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeTbCmpCmpResult(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/%s/cmp_result/", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 表比较-api 启动比较
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs describeTbCmpStart(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/active/tb_cmp/%s/start/", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 对象比较 - 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listObjCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 对象比较 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public I2Rs.I2CreateRs createObjCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 对象比较 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteObjCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 对象比较 - 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeObjCmp(String uuid) throws I2softException {
String url = String.format("%s/active/obj_cmp/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 对象比较 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopObjCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 对象比较-比较结果时间列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listObjCmpResultTimeList(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp/result_time_list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 对象比较-比较任务结果
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeObjCmpResult(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp/result", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 获取对象比较状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listObjCmpStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp/status", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 对象比较-比较结果的删除
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs describeObjCmpResultTimeList(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp/result_time_list", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 对象比较-比较结果详细信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listObjCmpCmpInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp/cmp_info", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 对象比较 - 删除(Oracle菜单)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteOracleObjCmp(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_cmp/obj_cmp_oracle", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 对象修复 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public I2Rs.I2CreateRs createObjFix(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_fix", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 对象修复 - 获取单个
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeObjFix(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/active/obj_fix/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 对象修复 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteObjFix(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_fix", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 对象修复 - 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listObjFix(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_fix", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 对象修复-操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs operateObjFix(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_fix/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 对象修复-操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs restartObjFix(StringMap args) throws I2softException {
args.put("operate", "restart");
String url = String.format("%s/active/obj_fix/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 对象修复-操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopObjFix(StringMap args) throws I2softException {
args.put("operate", "stop");
String url = String.format("%s/active/obj_fix/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 对象修复 - 修复结果
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeObjFixResult(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_fix/result", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 对象修复--获取状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listObjFixStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/obj_fix/status", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 备端接管-获取网卡列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listBkTakeoveNetworkCard(StringMap args) throws I2softException {
String url = String.format("%s/active/bk_takeover/bk_network_card", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 备端接管-新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public I2Rs.I2CreateRs createBkTakeover(StringMap args) throws I2softException {
String url = String.format("%s/active/bk_takeover", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 备端接管-查看
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeBkTakeover(String uuid) throws I2softException {
String url = String.format("%s/active/bk_takeover/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 备机接管-删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteBkTakeover(StringMap args) throws I2softException {
String url = String.format("%s/active/bk_takeover", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 备机接管-接管结果
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeBkTakeoverResult(StringMap args) throws I2softException {
String url = String.format("%s/active/bk_takeover/result", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 备机接管-操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopBkTakeover(StringMap args) throws I2softException {
args.put("operate", "stop");
String url = String.format("%s/active/bk_takeover/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 备机接管-操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs restartBkTakeover(StringMap args) throws I2softException {
args.put("operate", "restart");
String url = String.format("%s/active/bk_takeover/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 备端接管-获取状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listBkTakeoverStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/bk_takeover/status", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 备端接管列表
*
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listBkTakeover() throws I2softException {
String url = String.format("%s/active/bk_takeover", auth.cc_url);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 反向规则-新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public I2Rs.I2CreateRs createReverse(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2CreateRs.class);
}
/**
* 反向规则-删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteReverse(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 反向规则-获取单个规则信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeReverse(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse/rule_single", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 反向规则-获取列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listReverse(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 反向规则-状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listReverseStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 反向规则-停止
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopReverse(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse/stop", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 反向规则-重启反向任务
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs restartReverse(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse/restart", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 反向规则-查看
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSingleReverse(StringMap args) throws I2softException {
String url = String.format("%s/active/reverse", auth.cc_url);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 通用操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map syncRuleCommonOperate(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/common_operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 通用状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listSyncRulesGeneralStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/general_status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 装载信息流量图
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRulesLoadInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/load_info", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 流量图
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRulesMrtg(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/mrtg", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 已同步表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listRuleSyncTable(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/sync_table", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 已同步的对象
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRulesHasSync(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/sync_obj", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 已同步的对象具体信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRulesObjInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/sync_obj_info", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 同步失败的对象
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRulesFailObj(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/fail_obj", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 增量失败DDL
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRulesIncreDdl(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/incre_ddl", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 增量失败DML
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listRuleIncreDml(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/incre_dml", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 已同步的对象具体信息(DML解析)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeExtractSyncRulesObjInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/extract_sync_obj_info", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 已同步的对象具体信息(DML装载)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeLoadSyncRulesObjInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/load_sync_obj_info", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 增量失败DML统计
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSyncRulesDML(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/incre_dml_summary", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 增量失败统计删除(失败对象)
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs deleteSyncRulesDML(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/incre_dml_summary", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 同步规则 - 增量失败DML统计 - 表修复
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map increDmlFixAll(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/table_fix_all", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 选择表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeRuleZStructure(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/z_structure", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 数据库预检
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeRuleDbCheck(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/db_check", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 获取残留规则(废弃)
*
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeRuleGetFalseRule() throws I2softException {
String url = String.format("%s/active/rule/get_false_rule", auth.cc_url);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 同步规则 - 装载延迟统计报表(废弃)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listRuleLoadDelayReport(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/load_delay_report", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 装载统计报表(废弃)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listRuleLoadReport(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/load_report", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则-日志下载(废弃)
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs downloadLog(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/log_download", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 同步规则-选择用户
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeRuleSelectUser(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/select_user", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 增量表DML抽取统计
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listIncreDmlExtract(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/incre_dml_extract", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 增量表DML装载统计
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listIncreDmlLoad(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/incre_dml_load", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 解析热点图
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listExtractHeatMap(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/extract_heat_map", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 装载热点图
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listLoadHeatMap(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/load_heat_map", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 同步规则 - 修改维护模式
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs switchActiveRuleMaintenance(StringMap args) throws I2softException {
String url = String.format("%s/active/rule/maintenance", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy