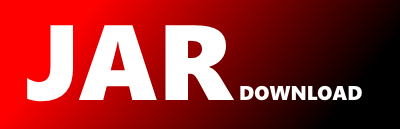
com.i2soft.cdm.v20220622.Cdm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2up-java-sdk Show documentation
Show all versions of i2up-java-sdk Show documentation
Information2 United Data Management Platform SDK for Java
The newest version!
package com.i2soft.cdm.v20220622;
import com.i2soft.common.Auth;
import com.i2soft.http.I2Rs;
import com.i2soft.http.I2softException;
import com.i2soft.http.Response;
import com.i2soft.util.StringMap;
import java.util.Map;
public final class Cdm {
/**
* Auth 对象
*/
private final Auth auth;
/**
* 构建一个新对象
*
* @param auth Auth对象
*/
public Cdm(Auth auth) {
this.auth = auth;
}
/**
* 自动演练规则 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createCdmDrill(StringMap args) throws I2softException {
String url = String.format("%s/cdm_drill", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 自动演练规则 - 获取组
*
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeCdmDrill(String uuid) throws I2softException {
String url = String.format("%s/cdm_drill/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 自动演练规则 - 获取组
*
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeCdmDrillGroup(String uuid) throws I2softException {
String url = String.format("%s/vp/drill/group/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 自动演练规则 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteCdmDrill(StringMap args) throws I2softException {
String url = String.format("%s/cdm_drill", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 自动演练规则 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listCdmDrillStatus(StringMap args) throws I2softException {
String url = String.format("%s/cdm_drill/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 自动演练规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map operateCdm(StringMap args) throws I2softException {
String url = String.format("%s/cdm_drill/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 整机复制 --- 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createCdm(StringMap args) throws I2softException {
String url = String.format("%s/cdm", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 整机复制 --- 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeCdm(String uuid) throws I2softException {
String url = String.format("%s/cdm/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 整机复制 --- 修改
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs describeCdm(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/cdm/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 整机复制 --- 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listCdm(StringMap args) throws I2softException {
String url = String.format("%s/cdm", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 整机复制 --- 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listCdmStatus(StringMap args) throws I2softException {
String url = String.format("%s/cdm/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 整机复制 --- 根据工作机获取规则
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getByWk(StringMap args) throws I2softException {
String url = String.format("%s/cdm/get_by_wk", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 备份点列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getPointList(StringMap args) throws I2softException {
String url = String.format("%s/cdm/point_full_info_list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 获取网卡列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getNetworkList(StringMap args) throws I2softException {
String url = String.format("%s/cdm/network_list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeFfomount(String uuid) throws I2softException {
String url = String.format("%s/ffo_mount/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 根据存储获取工作机列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getNodeList(StringMap args) throws I2softException {
String url = String.format("%s/cdm/restore_node_list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 获取资源列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getResourceList(StringMap args) throws I2softException {
String url = String.format("%s/cdm/drp_list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 获取主机存储资源
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getHostStorageList(StringMap args) throws I2softException {
String url = String.format("%s/cdm/host_storage_list", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 按虚机恢复获取磁盘
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getVmInfo(StringMap args) throws I2softException {
String url = String.format("%s/cdm/vm_info", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 整机恢复 --- 新建
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs createCdmRecovery(StringMap args) throws I2softException {
String url = String.format("%s/cdm_recovery", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 整机恢复 --- 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs operateCdmRecovery(StringMap args) throws I2softException {
String url = String.format("%s/cdm_recovery/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 整机恢复 --- 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listCdmRecovery(StringMap args) throws I2softException {
String url = String.format("%s/cdm_recovery", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 整机恢复 --- 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listCdmRecoveryStatus(StringMap args) throws I2softException {
String url = String.format("%s/cdm_recovery/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 接管/演练 --- 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map takeOverDrillList(StringMap args) throws I2softException {
String url = String.format("%s/cdm_rule", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 接管/演练 --- 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createTakeOverDrill(StringMap args) throws I2softException {
String url = String.format("%s/cdm_rule", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 接管/演练 --- 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteTakeOverDrill(StringMap args) throws I2softException {
String url = String.format("%s/cdm_rule", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 接管/演练 --- 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeTakeOverDrill(String uuid) throws I2softException {
String url = String.format("%s/cdm_rule/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 接管/演练 --- 获取虚机状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getVmStatus(StringMap args) throws I2softException {
String url = String.format("%s/cdm_rule/vm_status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 接管/演练 --- 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map operateTakeOverDrill(StringMap args) throws I2softException {
String url = String.format("%s/cdm_rule/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createFfoMount(StringMap args) throws I2softException {
String url = String.format("%s/ffo_mount", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 修改
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyFfoMount(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/ffo_mount/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map ffoMountList(StringMap args) throws I2softException {
String url = String.format("%s/ffo_mount", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 获取状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listFfoMountStatus(StringMap args) throws I2softException {
String url = String.format("%s/ffo_mount/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteFfoMount(StringMap args) throws I2softException {
String url = String.format("%s/ffo_mount", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 环境检测 -- Oracle是否开启归档
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs verifyOracleArchiveMode(StringMap args) throws I2softException {
String url = String.format("%s/cdm/verify_oracle_archive_mode", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy