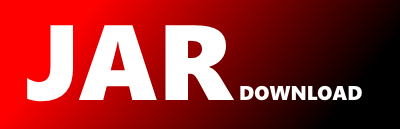
com.i2soft.resource.v20240819.ActiveNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2up-java-sdk Show documentation
Show all versions of i2up-java-sdk Show documentation
Information2 United Data Management Platform SDK for Java
The newest version!
package com.i2soft.resource.v20240819;
import com.i2soft.http.I2Req;
import com.i2soft.http.I2Rs;
import com.i2soft.http.I2softException;
import com.i2soft.http.Response;
import com.i2soft.common.Auth;
import com.i2soft.util.StringMap;
import java.util.Map;
public final class ActiveNode {
/**
* Auth 对象
*/
private final Auth auth;
/**
* 构建一个新对象
*
* @param auth Auth对象
*/
public ActiveNode(Auth auth) {
this.auth = auth;
}
/**
* 未激活机器节点列表
*
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listInactiveNodes() throws I2softException {
String url = String.format("%s/active/node/inactive_list", auth.cc_url);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 激活机器节点
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map activeNode(StringMap args) throws I2softException {
String url = String.format("%s/active/node", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 机器节点状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listNodeStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/node/status", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 机器节点列表(搜索)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listNodes(StringMap args) throws I2softException {
String url = String.format("%s/active/node", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 机器节点详细信息
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map descriptNode(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/active/node/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 状态信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map descriptNodeDebugInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/node/debug_info", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 修改机器节点
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyNode(StringMap args) throws I2softException {
String url = String.format("%s/active/node", auth.cc_url);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 删除机器节点
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteNode(StringMap args) throws I2softException {
String url = String.format("%s/active/node", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 机器节点升级
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs upgradeNode(StringMap args) throws I2softException {
String url = String.format("%s/active/node/upgrade", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 机器节点升级副本
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs renewActiveNode(StringMap args) throws I2softException {
String url = String.format("%s/active/node/renew", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 机器节点-维护模式切换
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs switchMaintenance(StringMap args) throws I2softException {
String url = String.format("%s/active/node/maintenance", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 获取字符集
*
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getCharset() throws I2softException {
String url = String.format("%s/active/db/charset", auth.cc_url);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 库节点 - 维护模式切换
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs switchDbMaintenance(StringMap args) throws I2softException {
String url = String.format("%s/active/db/maintenance", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 库节点列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listDbs(StringMap args) throws I2softException {
String url = String.format("%s/active/db", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 测试数据库连接(7.1.75 )
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map checkDbLink(StringMap args) throws I2softException {
String url = String.format("%s/active/db/db_check", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 库节点状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listDbStatus(StringMap args) throws I2softException {
String url = String.format("%s/active/db/status", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 库节点-新建 (格式统一7.1.75 )
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createDbUnified(StringMap args) throws I2softException {
String url = String.format("%s/active/db", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 库节点 - 修改
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyDb(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/active/db/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 表空间查询接口
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs describeDbSpace(StringMap args) throws I2softException {
String url = String.format("%s/active/db/space_query", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 删除库节点
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteDb(StringMap args) throws I2softException {
String url = String.format("%s/active/db", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 库节点 - 批量导入
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs batchCreateDbs(StringMap args) throws I2softException {
String url = String.format("%s/active/db/batch", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 机器节点 - 批量导入
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs batchCreateActiveNodes(StringMap args) throws I2softException {
String url = String.format("%s/active/node/batch", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 单个库节点信息
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeDb(String uuid) throws I2softException {
String url = String.format("%s/active/db/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 重新生成
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs rebuildActiveNode(StringMap args) throws I2softException {
String url = String.format("%s/active/node/rebuild", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 刷新
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map refresgActiveNode(StringMap args) throws I2softException {
String url = String.format("%s/active/node/refresh", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 机器节点 - 重启进程
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map restartAllProcess(StringMap args) throws I2softException {
String url = String.format("%s/active/node/process_restart", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 库节点 - 身份认证信息
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getActiveDbAuthInfo(StringMap args) throws I2softException {
String url = String.format("%s/active/db/auth_info", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 批量新建(sqlserver)
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs batchCreateSqlserverDbs(StringMap args) throws I2softException {
String url = String.format("%s/active/db/db_batch", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy