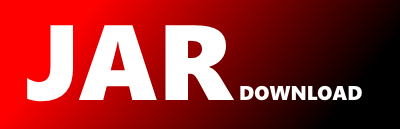
com.i2soft.vp.v20240819.VirtualizationSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2up-java-sdk Show documentation
Show all versions of i2up-java-sdk Show documentation
Information2 United Data Management Platform SDK for Java
The newest version!
package com.i2soft.vp.v20240819;
import com.i2soft.http.I2Req;
import com.i2soft.http.I2Rs;
import com.i2soft.http.I2softException;
import com.i2soft.http.Response;
import com.i2soft.common.Auth;
import com.i2soft.util.StringMap;
import java.util.Map;
public final class VirtualizationSupport {
/**
* Auth 对象
*/
private final Auth auth;
/**
* 构建一个新对象
*
* @param auth Auth对象
*/
public VirtualizationSupport(Auth auth) {
this.auth = auth;
}
/**
* Dashboard - 虚机规则 成功率
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpRuleRate(StringMap args) throws I2softException {
String url = String.format("%s/dashboard/vp_rule", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* Dashboard - 虚机 保护率
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVmProtectRate(StringMap args) throws I2softException {
String url = String.format("%s/dashboard/vp_vm", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createVpBackup(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 修改
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyVpBackup(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/backup/group/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpBackup(String uuid) throws I2softException {
String url = String.format("%s/vp/backup/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 虚机备份 - 获取单个(组)
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpBackupGroup(String uuid) throws I2softException {
String url = String.format("%s/vp/backup/group/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 虚机备份 - 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpBackup(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 列表(组)
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpBackupGroup(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup/group", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpBackupStatus(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 操作 启停
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map startVpBackup(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 操作 启停
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map stopVpBackup(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteVpBackup(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 虚机备份 - 删除备份点
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs deleteVpBackupPoint(StringMap args) throws I2softException {
String url = String.format("%s/vp/backup/backup_data", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机恢复 - 新建
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs createVpRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/recovery", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机恢复 - 获取单个 组
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpRecoveryGroup(String uuid) throws I2softException {
String url = String.format("%s/vp/recovery/group/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 虚机恢复 - 获取列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/recovery", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机恢复 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpRecoveryStatus(StringMap args) throws I2softException {
String url = String.format("%s/vp/recovery/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机恢复 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs startVpRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/recovery/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机恢复 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopVpRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/recovery/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机恢复 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs clearFinishVpRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/recovery/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机恢复 - 删除
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs deleteVpRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/recovery", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移/复制 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createVpMove(StringMap args) throws I2softException {
String url = String.format("%s/vp/move", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 虚机复制 - 批量创建
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs batchCreateVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/batch", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机复制 - 修改
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyVpRepGroup(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/group/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpMove(String uuid) throws I2softException {
String url = String.format("%s/vp/move/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpRep(String uuid) throws I2softException {
String url = String.format("%s/vp/rep/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 修改模板
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyVpMove(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/move/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 修改模板
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map modifyVpRep(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 获取列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpMove(StringMap args) throws I2softException {
String url = String.format("%s/vp/move", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 获取列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpMoveStatus(StringMap args) throws I2softException {
String url = String.format("%s/vp/move/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpRepStatus(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopVpMove(StringMap args) throws I2softException {
String url = String.format("%s/vp/move/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs startVpMove(StringMap args) throws I2softException {
String url = String.format("%s/vp/move/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs moveVpMove(StringMap args) throws I2softException {
String url = String.format("%s/vp/move/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs finishVpMove(StringMap args) throws I2softException {
String url = String.format("%s/vp/move/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs createTargeVm(StringMap args) throws I2softException {
String url = String.format("%s/vp/move/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机复制 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs stopVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机复制 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs startVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机复制 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs failoverVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机复制 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs failbackVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机复制 - 操作
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs startImmediatelyVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移/复制 - 删除
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs deleteVpMove(StringMap args) throws I2softException {
String url = String.format("%s/vp/move", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移/复制 - 删除
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs deleteVpRep(StringMap args) throws I2softException {
String url = String.format("%s/vp/rep", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 虚机迁移/复制 - 获取快照
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpRepPointList(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/%s/point_list", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机迁移/复制 - 获取快照
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listMovePointList(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/%s/point_list", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 虚机复制 - 获取快照详情(备份中心)
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeSnapshotInfo(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/rep/%s/snapshot_info", auth.cc_url, uuid);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpDrill(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 新建
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map createVpDrill(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 获取单个(组)
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpDrill(String uuid) throws I2softException {
String url = String.format("%s/vp/drill/group/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 演练规则 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteVpDrill(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpDrillStatus(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 获取控制台地址
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map getConsoleUrl(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill/console_url", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map stopVpDrill(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map startVpDrill(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 演练规则 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map setStatusVpDrill(StringMap args) throws I2softException {
String url = String.format("%s/vp/drill/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 文件恢复 - 新建
*
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs createVpFileRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/file_recovery", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 文件恢复 - 修改
*
* @param uuid: uuid
* @param args: 参数详见 API 手册
* @return code, message
* @throws I2softException:
*/
public I2Rs.I2SmpRs modifyVpFileRecovery(String uuid, StringMap args) throws I2softException {
String url = String.format("%s/vp/file_recovery/%s", auth.cc_url, uuid);
Response r = auth.client.put(url, args);
return r.jsonToObject(I2Rs.I2SmpRs.class);
}
/**
* 文件恢复 - 列表
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpFileRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/file_recovery", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 文件恢复 - 获取单个
*
* @param uuid: uuid
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map describeVpFileRecovery(String uuid) throws I2softException {
String url = String.format("%s/vp/file_recovery/%s", auth.cc_url, uuid);
Response r = auth.client.get(url, new StringMap());
return r.jsonToMap();
}
/**
* 文件恢复 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map attachVpFileRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/file_recovery/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 文件恢复 - 操作
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map detachVpFileRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/file_recovery/operate", auth.cc_url);
Response r = auth.client.post(url, args);
return r.jsonToMap();
}
/**
* 文件恢复 - 状态
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map listVpFileRecoveryStatus(StringMap args) throws I2softException {
String url = String.format("%s/vp/file_recovery/status", auth.cc_url);
Response r = auth.client.get(url, args);
return r.jsonToMap();
}
/**
* 文件恢复 - 删除
*
* @param args: 参数详见 API 手册
* @return 参数详见 API 手册
* @throws I2softException:
*/
public Map deleteVpFileRecovery(StringMap args) throws I2softException {
String url = String.format("%s/vp/file_recovery", auth.cc_url);
Response r = auth.client.delete(url, args);
return r.jsonToMap();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy